替换IOUtils.copy(inputStream, response.getOutputStream());方法
时间: 2023-12-27 14:06:27 浏览: 61
可以使用Java 7中提供的try-with-resources语句来自动关闭输入输出流,同时使用Apache Commons IO库中的IOUtils.copyLarge方法来复制数据。
这里是一个示例代码:
```java
try (InputStream inputStream = new FileInputStream(file)) {
OutputStream outputStream = response.getOutputStream();
IOUtils.copyLarge(inputStream, outputStream);
} catch (IOException e) {
// 处理异常
}
```
需要注意的是,如果使用try-with-resources语句,则无需手动关闭输入输出流。同时,如果要复制的数据量较大,建议使用IOUtils.copyLarge方法,以避免内存溢出。
相关问题
java 使用response.getOutputStream()添加一层文件夹下载文件
要实现在 Java 中使用 `response.getOutputStream()` 下载文件并添加一层文件夹,可以按照以下步骤进行:
1. 设置响应头
在使用 `response.getOutputStream()` 下载文件时,需要设置响应头,包括文件名、文件类型等信息。在这里,我们需要设置一个 Content-Disposition 头部,用于指定浏览器下载文件时的文件名和保存方式。同时,我们可以在文件名前添加一个文件夹名称,以实现添加一层文件夹的效果。示例代码如下:
```
String fileName = "example.txt";
String folderName = "downloads";
String contentType = "application/octet-stream";
response.setHeader("Content-Disposition", "attachment;filename=" + folderName + "/" + fileName);
response.setContentType(contentType);
```
2. 获取文件流
接下来,我们需要获取要下载的文件的输入流。在这里,我们使用 `FileInputStream` 类来获取文件流。示例代码如下:
```
String filePath = "example.txt";
File file = new File(filePath);
InputStream inputStream = new FileInputStream(file);
```
3. 写入响应流
最后,我们将文件流写入响应流中,以实现下载文件的功能。在这里,我们使用 `ServletOutputStream` 类来获取响应流,并使用 `IOUtils` 类将文件流写入响应流中。示例代码如下:
```
ServletOutputStream outputStream = response.getOutputStream();
IOUtils.copy(inputStream, outputStream);
outputStream.flush();
outputStream.close();
inputStream.close();
```
以上代码将在浏览器下载一个名为 `downloads/example.txt` 的文件,其中 `example.txt` 是要下载的文件名,`downloads` 是添加的文件夹名称。
优化代码@PostMapping("/downInvoices4") public void downInvoices4(@RequestBody List<String> pathList,HttpServletResponse response) { String UUIDStr = String.valueOf(UUID.randomUUID()); String folderName = "invoice" + UUIDStr.replace("-",""); response.setContentType("application/octet-stream"); response.setHeader("Content-Disposition", "attachment;filename="+folderName+".zip"); try { URL url = new URL(pathList.get(0)); URLConnection coon = url.openConnection(); InputStream is = coon.getInputStream(); ServletOutputStream sos = response.getOutputStream(); ZipInputStream zis = new ZipInputStream(is); ZipEntry zipEntry =zis.getNextEntry(); ZipOutputStream zos = new ZipOutputStream(sos); zos.putNextEntry(zipEntry); zos.closeEntry(); IoUtils.copy(zis,zos); zos.close(); zis.close(); sos.close(); is.close(); } catch (Exception e) { throw new RuntimeException(e); } }
这段代码可以进行一些优化:
1. 首先,建议将该方法的返回类型改为 ResponseEntity<byte[]>,这样可以更好地控制响应的内容。同时,也可以避免一些潜在的问题,比如下载文件时可能会出现乱码的情况。
2. 接着,可以将生成文件名和设置响应头的代码提取出来,作为一个单独的方法。这样可以提高代码的可读性和复用性。
3. 然后,可以使用 try-with-resources 语句来自动关闭流,避免出现资源泄漏的情况。
4. 最后,建议对输入参数进行必要的校验,比如判断 pathList 是否为空,以及其中是否包含有效的 URL。
下面是优化后的代码示例:
```
@PostMapping("/downInvoices4")
public ResponseEntity<byte[]> downInvoices4(@RequestBody List<String> pathList) {
validate(pathList); // 校验输入参数
try {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ZipOutputStream zos = new ZipOutputStream(baos);
for (String path : pathList) {
URL url = new URL(path);
URLConnection conn = url.openConnection();
InputStream is = conn.getInputStream();
ZipEntry entry = new ZipEntry(getFileName(path));
zos.putNextEntry(entry);
IoUtils.copy(is, zos);
zos.closeEntry();
is.close();
}
zos.close();
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDisposition(ContentDisposition.attachment().filename(getZipFileName()).build());
return new ResponseEntity<>(baos.toByteArray(), headers, HttpStatus.OK);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
private void validate(List<String> pathList) {
if (pathList == null || pathList.isEmpty()) {
throw new IllegalArgumentException("pathList cannot be null or empty");
}
for (String path : pathList) {
try {
new URL(path);
} catch (MalformedURLException e) {
throw new IllegalArgumentException("invalid URL: " + path);
}
}
}
private String getFileName(String path) {
int index = path.lastIndexOf("/");
return index >= 0 ? path.substring(index + 1) : path;
}
private String getZipFileName() {
String UUIDStr = String.valueOf(UUID.randomUUID());
String folderName = "invoice" + UUIDStr.replace("-", "");
return folderName + ".zip";
}
```
相关推荐
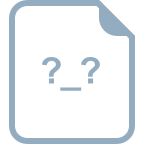
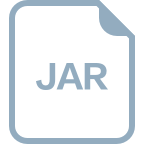
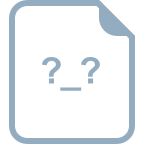












