综合利用Swing界面和自己写音乐播放类,设计音乐播放器如下:(1)播放界面插入已有图片,纯Swing给定的组件可以设计出一个普通的音乐播放器界面。(2)音乐播放方面,不能调用其他第三方jar包,只能用JDK底层自带的API类,例如JavaSound。使用JavaSound API,可以实现各种基于声音的应用,例如声音录制、音乐播放、网络电话、音乐编辑等。JavaSound API又以各种解码和合成器SPI(服务提供者接口, Service Provider Interface)为基础,实现各种音乐格式的解码与转码。 给出java代码
时间: 2024-03-20 21:39:15 浏览: 15
以下是一个简单的音乐播放器的Java代码示例,实现了播放、暂停、停止等功能,并且插入了已有的图片作为播放器界面的背景:
```java
import javax.sound.sampled.*;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.IOException;
public class MusicPlayer extends JFrame implements ActionListener {
private JButton playBtn, pauseBtn, stopBtn;
private JLabel timeLabel, durationLabel;
private JSlider progressSlider;
private Clip clip;
private Timer timer;
private long clipTime;
public MusicPlayer() {
super("Music Player");
// 设置播放器界面背景
ImageIcon bgImage = new ImageIcon("music_bg.jpg");
JLabel bgLabel = new JLabel(bgImage);
bgLabel.setBounds(0, 0, bgImage.getIconWidth(), bgImage.getIconHeight());
this.getLayeredPane().add(bgLabel, new Integer(Integer.MIN_VALUE));
// 创建控制按钮
playBtn = new JButton("Play");
playBtn.addActionListener(this);
pauseBtn = new JButton("Pause");
pauseBtn.addActionListener(this);
stopBtn = new JButton("Stop");
stopBtn.addActionListener(this);
// 创建时间和进度条显示标签
timeLabel = new JLabel("00:00");
durationLabel = new JLabel("00:00");
progressSlider = new JSlider(0, 0, 0);
// 创建播放器布局
JPanel controlPanel = new JPanel(new GridLayout(1, 3, 10, 0));
controlPanel.add(playBtn);
controlPanel.add(pauseBtn);
controlPanel.add(stopBtn);
JPanel progressPanel = new JPanel(new BorderLayout());
progressPanel.add(timeLabel, BorderLayout.WEST);
progressPanel.add(progressSlider, BorderLayout.CENTER);
progressPanel.add(durationLabel, BorderLayout.EAST);
JPanel playerPanel = new JPanel(new BorderLayout());
playerPanel.add(controlPanel, BorderLayout.NORTH);
playerPanel.add(progressPanel, BorderLayout.CENTER);
this.getContentPane().add(playerPanel);
this.setSize(bgImage.getIconWidth(), bgImage.getIconHeight());
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setVisible(true);
}
public static void main(String[] args) {
MusicPlayer player = new MusicPlayer();
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == playBtn) {
if (clip == null) {
try {
File file = new File("music.mp3");
AudioInputStream audioInputStream = AudioSystem.getAudioInputStream(file);
AudioFormat format = audioInputStream.getFormat();
DataLine.Info info = new DataLine.Info(Clip.class, format);
clip = (Clip) AudioSystem.getLine(info);
clip.open(audioInputStream);
clipTime = clip.getMicrosecondPosition();
progressSlider.setMaximum((int) clip.getMicrosecondLength() / 1000000);
durationLabel.setText(formatTime(clip.getMicrosecondLength() / 1000));
} catch (UnsupportedAudioFileException ex) {
ex.printStackTrace();
} catch (LineUnavailableException ex) {
ex.printStackTrace();
} catch (IOException ex) {
ex.printStackTrace();
}
}
if (!clip.isActive()) {
clip.setMicrosecondPosition(clipTime);
}
clip.start();
// 启动定时器,更新进度条和时间显示标签
timer = new Timer(500, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (clip != null) {
long currentTime = clip.getMicrosecondPosition();
clipTime = currentTime;
progressSlider.setValue((int) currentTime / 1000000);
timeLabel.setText(formatTime(currentTime / 1000));
}
}
});
timer.start();
} else if (e.getSource() == pauseBtn) {
if (clip != null) {
clipTime = clip.getMicrosecondPosition();
clip.stop();
timer.stop();
}
} else if (e.getSource() == stopBtn) {
if (clip != null) {
clip.stop();
clipTime = 0;
clip.setMicrosecondPosition(clipTime);
progressSlider.setValue(0);
timeLabel.setText("00:00");
timer.stop();
}
}
}
// 将毫秒数格式化为00:00的形式
private String formatTime(long milliseconds) {
long seconds = milliseconds / 1000;
long minutes = seconds / 60;
seconds = seconds % 60;
return String.format("%02d:%02d", minutes, seconds);
}
}
```
在上述代码中,我们使用了JFrame和Swing组件来创建了一个音乐播放器的界面,并且插入了已有的图片作为背景。我们使用了JavaSound API中的Clip类来实现音乐的播放功能,并且使用了JSlider和JLabel等组件来显示播放进度和时间。
在播放按钮被点击时,我们首先读取音频文件并使用Clip类来播放音频数据。同时,我们启动一个定时器来定时更新进度条和时间显示标签的值,实现了进度条的自动更新。在暂停和停止按钮被点击时,我们暂停或停止Clip对象的播放,并且停止定时器的运行。
需要注意的是,本示例代码只支持播放MP3格式的音频文件。如果需要支持其他格式的音频文件,需要针对不同的格式进行解码和转码。
相关推荐
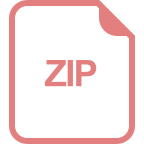
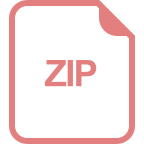














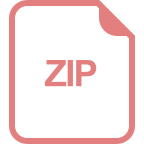
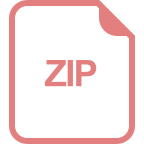