Intent deleteIntent = new Intent(this, MainActivity.class); deleteIntent.setAction(ACTION_DELETE_NOTIFICATION); PendingIntent pendingDeleteIntent = PendingIntent.getBroadcast( this, 0, deleteIntent, PendingIntent.FLAG_UPDATE_CURRENT | PendingIntent.FLAG_IMMUTABLE); if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) { // Create the NotificationChannel CharSequence name = "My App Notification Channel"; String description = "My App Notifications"; int importance = NotificationManager.IMPORTANCE_DEFAULT; NotificationChannel channel = new NotificationChannel("com.example.myapplication", name, importance); channel.setDescription(description); // Register the channel with the system; you can't change the importance // or other notification behaviors after this NotificationManager notificationManager = getSystemService(NotificationManager.class); notificationManager.createNotificationChannel(channel); } Notification notification = new NotificationCompat.Builder(this, "com.example.myapplication") .setSmallIcon(R.drawable.ic_launcher_background) .setContentTitle("My notification") .setContentText("Hello World!!!!") .addAction(R.drawable.ic_launcher_foreground, "Delete", pendingDeleteIntent) .build(); NotificationManagerCompat.from(this).notify(CHANNEL_CHARGING_NOTIFICATION_ID, notification);为什么action收不到
时间: 2024-01-12 20:02:51 浏览: 24
可能是因为你没有在BroadcastReceiver中正确地处理ACTION_DELETE_NOTIFICATION这个action。请确认你的BroadcastReceiver中能够正确地接收并处理这个action,例如:
```
public class MyBroadcastReceiver extends BroadcastReceiver {
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (action != null && action.equals(ACTION_DELETE_NOTIFICATION)) {
// 在这里处理删除通知的逻辑
// ...
}
}
}
```
如果你已经正确地处理了ACTION_DELETE_NOTIFICATION这个action,但仍然无法收到通知删除的事件,请提供更多的代码和详细的错误信息,以便我们更好地帮助你解决问题。
相关问题
Intent deleteIntent = new Intent(this, MainActivity.class); deleteIntent.setAction(ACTION_DELETE_NOTIFICATION); PendingIntent pendingDeleteIntent = PendingIntent.getBroadcast(this, 0, deleteIntent, PendingIntent.FLAG_UPDATE_CURRENT | PendingIntent.FLAG_IMMUTABLE); NotificationCompat.Builder builder = new NotificationCompat.Builder(this, "com.example.myapplication") .setSmallIcon(R.drawable.ic_launcher_background) .setContentTitle("My notification") .setContentText("Hello World!") .addAction(R.drawable.ic_launcher_foreground, "Delete", pendingDeleteIntent); Notification build = builder.build(); NotificationManagerCompat.from(this).notify(CHANNEL_CHARGING_NOTIFICATION_ID, build); } 报错说 No Channel found for pkg=com.example.myapplication 如何解决
这个错误提示是因为在创建通知时使用了不存在的通知渠道,需要先创建一个通知渠道,然后将其与通知相关联。可以按照以下步骤解决:
1. 在应用的创建时,创建通知渠道,代码如下:
```java
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel channel = new NotificationChannel("com.example.myapplication", "MyApp", NotificationManager.IMPORTANCE_HIGH);
NotificationManager notificationManager = getSystemService(NotificationManager.class);
notificationManager.createNotificationChannel(channel);
}
```
2. 在创建通知时,指定通知渠道,代码如下:
```java
NotificationCompat.Builder builder = new NotificationCompat.Builder(this, "com.example.myapplication")
.setSmallIcon(R.drawable.ic_launcher_background)
.setContentTitle("My notification")
.setContentText("Hello World!")
.addAction(R.drawable.ic_launcher_foreground, "Delete", pendingDeleteIntent);
Notification build = builder.build();
NotificationManagerCompat.from(this).notify(CHANNEL_CHARGING_NOTIFICATION_ID, build);
```
这样就可以解决该错误。
Intent intent = new Intent(Welcome.this.MainActivity.class);中MainActivity.class报错怎么改
在这个 `Intent` 的构造函数中,第一个参数应该是一个 `Context` 对象,用于指定从哪个上下文(通常是一个活动)启动另一个活动。
您提供的代码 `Welcome.this.MainActivity.class` 中,`Welcome.this` 是一个 `Welcome` 活动的实例,而 `MainActivity.class` 是一个类对象,两者之间没有适当的联系。也就是说,您需要提供一个 `Context` 对象来替换 `Welcome.this`,以便正确地启动 `MainActivity`。
例如,如果您想从 `Welcome` 活动启动 `MainActivity`,您可以使用以下代码:
```
Intent intent = new Intent(Welcome.this, MainActivity.class);
startActivity(intent);
```
这里,`Welcome.this` 是 `Welcome` 活动的上下文,`MainActivity.class` 是 `MainActivity` 的类对象,`Intent` 构造函数使用这两个参数来创建一个新的 `Intent` 对象,并使用 `startActivity()` 方法启动 `MainActivity` 活动。
相关推荐
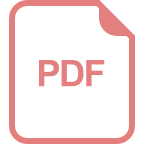
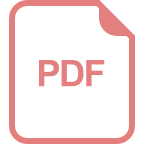













