java 一个实现mastermind的代码
时间: 2023-05-12 12:00:39 浏览: 235
Mastermind是一种经典游戏,用于猜测由几个位置和有限个数字组成的编码序列。Java是一种面向对象的编程语言,可以很容易地实现Mastermind的代码。下面是一个实现Mastermind的Java代码:
import java.util.*;
public class Mastermind {
private static final int CODE_LENGTH = 4;
private static final int MAX_GUESSES = 12;
private static final int NUM_COLORS = 6;
private static final String[] COLORS = {"R", "G", "B", "Y", "O", "P"};
private String secretCode;
private int numGuesses;
public Mastermind() {
secretCode = generateSecretCode();
numGuesses = 0;
}
public void play() {
Scanner input = new Scanner(System.in);
System.out.println("Welcome to Mastermind!");
while (numGuesses < MAX_GUESSES) {
System.out.print("Enter your guess (e.g. RBGY): ");
String guess = input.nextLine();
if (guess.length() != CODE_LENGTH) {
System.out.println("Invalid guess length! Must be " + CODE_LENGTH + " characters.");
continue;
}
if (!isValid(guess)) {
System.out.println("Invalid guess! Must consist of the characters R, G, B, Y, O, P.");
continue;
}
String result = checkGuess(guess);
if (result.equals("BBBB")) {
System.out.println("You win!");
return;
} else {
System.out.println("Result: " + result);
numGuesses++;
}
}
System.out.println("You lose! The secret code was " + secretCode + ".");
}
private String generateSecretCode() {
Random random = new Random();
StringBuilder code = new StringBuilder();
for (int i = 0; i < CODE_LENGTH; i++) {
int index = random.nextInt(NUM_COLORS);
code.append(COLORS[index]);
}
return code.toString();
}
private boolean isValid(String guess) {
for (char ch : guess.toCharArray()) {
if (ch < 'A' || ch > 'Z' || ch == 'F' || ch == 'I' || ch == 'J' || ch == 'K' || ch == 'N' || ch == 'Q' || ch == 'U' || ch == 'V' || ch == 'W' || ch == 'X' || ch == 'Z') {
return false;
}
}
return true;
}
private String checkGuess(String guess) {
StringBuilder result = new StringBuilder();
for (int i = 0; i < CODE_LENGTH; i++) {
char ch = guess.charAt(i);
if (secretCode.charAt(i) == ch) {
result.append('B');
} else if (secretCode.contains(Character.toString(ch))) {
result.append('W');
} else {
result.append('.');
}
}
return result.toString();
}
public static void main(String[] args) {
Mastermind game = new Mastermind();
game.play();
}
}
这个代码使用了很多常量和数组来表示游戏规则和颜色选项。generateSecretCode方法随机生成一个大小为CODE_LENGTH的编码序列。isValid方法检查猜测是否有效。checkGuess方法返回一个字符串,用B表示正确位置的正确数字,用W表示包含正确数字但位置不正确的数字,用.表示没有正确的数字。主方法创建一个Mastermind对象并开始游戏。如果用户成功猜到编码序列,游戏结束并输出“你赢了!”。如果用户达到MAX_GUESSES次猜测但未猜对,游戏结束并输出“你输了!”。
阅读全文
相关推荐
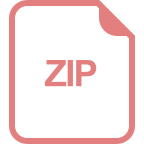
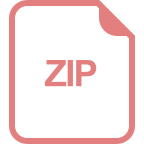
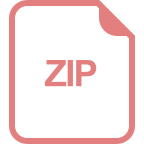
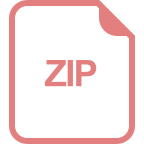
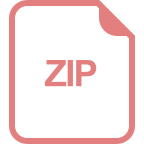
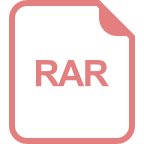
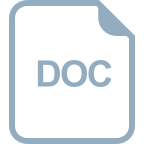
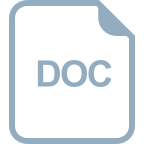
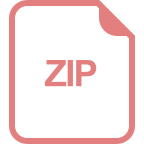
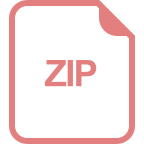
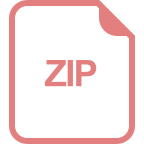
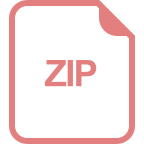
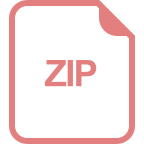
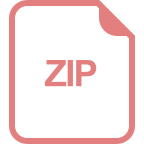
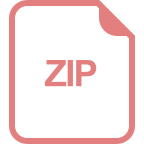
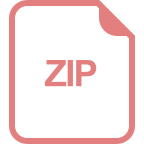
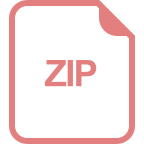
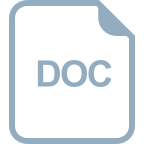
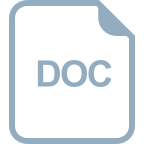