计算机图形学矩阵变换c++
时间: 2023-10-24 12:09:58 浏览: 165
计机图形学中,矩阵变换是一种常用的方法,用于对图形对象进行平移、旋转、缩放等操作。在给定的代码实现中,move函数用于实现矩阵的转置操作。转置操作是将矩阵的行和列交换位置,即将矩阵的第i行和第j列的元素互换。通过这样的操作,可以实现矩阵的行变为列,列变为行的效果。
矩阵变换在计算机图形学中有广泛的应用,如平移变换可以改变图形对象的位置,旋转变换可以改变图形对象的方向等。通过矩阵变换,可以对图形对象进行各种复杂的变换操作,从而实现图形的动态效果。
相关问题
计算机图形学图形变换
### 计算机图形学中的图形变换概念
在计算机图形学中,图形变换是指通过特定算法改变图像位置、大小或方向的过程。这些变换可以分为基本几何变换和平面投影变换两大类。对于二维图形而言,主要涉及平移、比例(缩放)、旋转三种基础变换。
#### 平移变换
平移操作用于移动对象而不改变其形状和尺寸。为了表示这种位移,在齐次坐标系下,任何一点 \( V = (x, y, 1) \)[^2] 可以被转换成新的位置:
\[ T(a,b)=\begin{pmatrix} 1 & 0 & a \\ 0& 1 & b\\ 0& 0& 1 \end{pmatrix}\]
其中 \(a\) 和 \(b\) 表示沿X轴和Y轴的方向上分别偏移的距离。应用此矩阵乘法即可完成平移效果[^1]。
```cpp
// C++ Code Example for Translation Transformation
void translate(float matrix[3][3], float tx, float ty){
// Initialize translation matrix with identity and add offsets at last column.
memset(matrix, 0, sizeof(matrix));
matrix[0][0] = 1; matrix[0][2] = tx;
matrix[1][1] = 1; matrix[1][2] = ty;
matrix[2][2] = 1;
}
```
```python
# Python Code Example for Translation Transformation
def translate(tx, ty):
"""Return the transformation matrix for translating by `tx` and `ty`.
Args:
tx (float): The amount to move along X-axis.
ty (float): The amount to move along Y-axis.
Returns:
list[list[float]]: A 3x3 homogeneous coordinate transform matrix."""
return [[1, 0, tx],
[0, 1, ty],
[0, 0, 1]]
```
#### 缩放变换
当需要调整物体的比例时,则采用缩放变换。该过程同样依赖于一个特殊的变换矩阵来放大或缩小目标对象。假设要沿着两个维度各自伸展至原来的s_x倍和s_y倍,则对应的变换矩阵如下所示:
\[ S(s_x,s_y)=\begin{pmatrix}s_x & 0 & 0 \\ 0&s_y & 0\\ 0& 0& 1 \end{pmatrix}\]
这会使得原点处的对象按照指定因子扩大或收缩。
```cpp
// C++ Code Example for Scaling Transformation
void scale(float matrix[3][3], float sx, float sy){
// Set up scaling factors on diagonal elements of an otherwise zeroed-out array.
memset(matrix, 0, sizeof(matrix));
matrix[0][0] = sx;
matrix[1][1] = sy;
matrix[2][2] = 1;
}
```
```python
# Python Code Example for Scaling Transformation
def scale(sx, sy):
"""Create a scaling transformation matrix that scales points around origin
Args:
sx (float): Scale factor applied horizontally.
sy (float): Scale factor applied vertically.
Returns:
list[list[float]]: A 3x3 homogenous coordinates transformation matrix"""
return [
[sx, 0 , 0 ],
[0 ,sy, 0 ],
[0 , 0 , 1 ]
]
```
#### 旋转变换
最后一种常见的变换方式就是绕着某个中心点进行角度θ度数的顺时针/逆时针转动。此时需要用到正弦函数sin()以及余弦cosine()计算新坐标的值,并构建相应的旋转矩阵R(θ):
\[ R(\theta )=\begin{pmatrix}\cos (\theta)&-\sin (\theta)&0\\\sin (\theta)&\cos (\theta)&0\\0&0&1\end{pmatrix}\]
上述公式能够帮助实现任意给定角度下的平面内旋转操作。
```cpp
// C++ Code Example for Rotation Transformation
void rotate(float matrix[3][3], double angle_degrees){
const double PI = acos(-1);
double radians = angle_degrees * PI / 180;
// Fill rotation matrix using sine/cosine values from given degree input converted into radian measure.
memset(matrix, 0, sizeof(matrix));
matrix[0][0] = cos(radians);
matrix[0][1] = -sin(radians);
matrix[1][0] = sin(radians);
matrix[1][1] = cos(radians);
matrix[2][2] = 1;
}
```
```python
import math
# Python Code Example for Rotation Transformation
def rotate(angle_degrees):
"""Generate a counterclockwise rotational transformation about z axis through 'angle' degrees
Args:
angle_degrees (int or float): Angle measured clockwise in degrees
Returns:
list[list[float]]: A 3x3 homogenous coordinates transformation matrix representing this operation."""
theta_rad = math.radians(angle_degrees)
c, s = round(math.cos(theta_rad), ndigits=6),round(math.sin(theta_rad),ndigits=6)
return [
[c,-s, 0],
[s,c, 0],
[0,0, 1]
]
```
计算机图形学使用c++如何编程通过矩阵运算实现视口变换
计算机图形学中,视口变换是一个关键步骤,它将模型空间的坐标转换到屏幕空间以便渲染。通常会使用4x4矩阵来表示这种变换,包括缩放、平移、旋转和平移操作。以下是使用C++和矩阵运算实现视口变换的基本步骤:
1. **建立基础矩阵**:
- **视口矩阵(View Matrix)**: 它描述了摄像机的位置和方向,使得对象看起来是从特定视角观察的。这个矩阵通常包含摄像机的位置、旋转和对齐信息。
2. **投影矩阵(Projection Matrix)**:
- **透视投影**:用于创建远近有深度感的效果,矩阵包含了焦距、视场角等参数。常见的有perspective()函数,其中包含一个fovy(视场垂直角度)、aspect ratio(宽高比)、zNear和zFar(近裁剪面和远裁剪面距离)。
3. **模型矩阵(Model Matrix)**:
- 当你想移动、旋转或缩放模型时,你需要构建该矩阵。这通常对应于物体在世界空间中的位置和变换。
4. **将变换组合成变换矩阵**:
- 将上述三个矩阵相乘得到最终的视口变换矩阵(View * Projection * Model),这是因为变换是顺序应用的,从最靠近摄像机的变换开始。
5. **应用变换**:
- 对每个三维顶点,将其乘以视口变换矩阵,将模型空间坐标转换为屏幕空间坐标。
以下是一个简化的示例代码片段:
```cpp
// 假设我们已经有了视口矩阵(view), 投影矩阵(proj)和模型矩阵(model)
glm::mat4 combinedTransform = proj * view * model;
// 顶点数据 (vertices) 为 glm::vec3 类型
for (const auto& vertex : vertices) {
glm::vec4 transformedVertex = combinedTransform * glm::vec4(vertex, 1.0f); // 透视除法,防止无限大坐标
// 接着处理屏幕空间坐标,如投射到窗口上
screenX = transformedVertex.x / transformedVertex.w;
screenY = transformedVertex.y / transformedVertex.w;
}
```
阅读全文
相关推荐
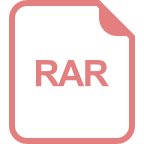

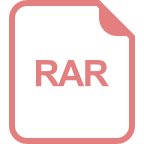










