js 用ES5写类的继承
时间: 2023-12-24 08:06:09 浏览: 67
使用ES5写类的继承可以通过以下方式:
1. 定义父类构造函数
```javascript
function Animal(name) {
this.name = name;
}
Animal.prototype.sayName = function() {
console.log(this.name);
}
```
2. 定义子类构造函数并继承父类
```javascript
function Dog(name, breed) {
// 调用父类构造函数
Animal.call(this, name);
this.breed = breed;
}
// 子类继承父类的原型方法
Dog.prototype = Object.create(Animal.prototype);
// 修复子类构造函数指向
Dog.prototype.constructor = Dog;
// 子类新增方法
Dog.prototype.bark = function() {
console.log('Woof!');
}
```
3. 创建子类实例并调用方法
```javascript
var myDog = new Dog('Buddy', 'Golden Retriever');
myDog.sayName(); // 输出 'Buddy'
myDog.bark(); // 输出 'Woof!'
```
相关问题
js 用es5写类的继承
// 父类
function Animal(name) {
this.name = name;
}
Animal.prototype.sayName = function() {
console.log('My name is ' + this.name);
};
// 子类
function Cat(name) {
Animal.call(this, name);
}
// 继承父类原型上的方法
Cat.prototype = Object.create(Animal.prototype);
// 修正 constructor 指向
Cat.prototype.constructor = Cat;
// 新增子类原型上的方法
Cat.prototype.sayMeow = function() {
console.log('Meow!');
};
// 实例化子类
var myCat = new Cat('Tom');
// 调用继承自父类的方法
myCat.sayName(); // 输出:"My name is Tom"
// 调用子类自己的方法
myCat.sayMeow(); // 输出:"Meow!"
用js es5的语法实现构造函数的继承
好的,以下是用 ES5 的语法实现构造函数的继承的例子:
```javascript
function Animal(name) {
this.name = name;
}
Animal.prototype.sayHello = function() {
console.log(`Hello, my name is ${this.name}.`);
}
function Dog(name, breed) {
Animal.call(this, name);
this.breed = breed;
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
Dog.prototype.sayBreed = function() {
console.log(`I am a ${this.breed} dog.`);
}
const fido = new Dog('Fido', 'Labrador Retriever');
fido.sayHello(); // Output: Hello, my name is Fido.
fido.sayBreed(); // Output: I am a Labrador Retriever dog.
```
在这个例子中,我们有两个构造函数:`Animal` 和 `Dog`。`Dog` 继承自 `Animal`。我们使用 `call` 方法在 `Dog` 构造函数中调用 `Animal` 构造函数,并传递 `this` 和 `name` 参数。然后,我们使用 `Object.create` 方法创建一个新对象,该对象的原型是 `Animal.prototype`,并将其分配给 `Dog.prototype`。最后,我们重置 `Dog.prototype.constructor` 属性以指向 `Dog` 构造函数,并添加一个新的 `sayBreed` 方法。最终,我们创建了一个 `Dog` 实例 `fido`,并调用了 `sayHello` 和 `sayBreed` 方法。
阅读全文
相关推荐
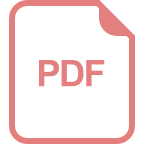
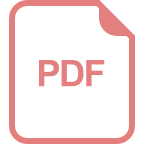
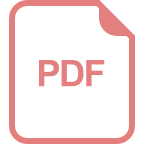













