linux 上 java 程序上传文件到 FTP
时间: 2024-02-25 22:57:15 浏览: 88
要在Linux上使用Java程序上传文件到FTP服务器,您可以使用Apache Commons Net库进行FTP操作。以下是一个简单的Java代码示例,用于将本地文件上传到FTP服务器:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.commons.net.ftp.FTP;
import org.apache.commons.net.ftp.FTPClient;
import org.apache.commons.net.ftp.FTPReply;
public class FTPUploader {
public static void main(String[] args) throws IOException {
String server = "ftp.example.com";
int port = 21;
String user = "ftpuser";
String pass = "ftppass";
FTPClient ftpClient = new FTPClient();
ftpClient.connect(server, port);
int replyCode = ftpClient.getReplyCode();
if (!FTPReply.isPositiveCompletion(replyCode)) {
ftpClient.disconnect();
System.err.println("FTP server refused connection.");
System.exit(1);
}
ftpClient.login(user, pass);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTP.BINARY_FILE_TYPE);
String localFile = "/path/to/local/file";
String remoteFile = "remote_file_name";
FileInputStream inputStream = new FileInputStream(new File(localFile));
boolean uploaded = ftpClient.storeFile(remoteFile, inputStream);
inputStream.close();
if (uploaded) {
System.out.println("Upload successful!");
} else {
System.out.println("Upload failed!");
}
ftpClient.logout();
ftpClient.disconnect();
}
}
```
在上面的代码中,您需要替换变量server,port,user和pass为您的FTP服务器的详细信息,然后指定本地文件路径和远程文件名以上传文件。
希望这些信息对您有所帮助。如果您还有其他问题,请随时问我。
阅读全文
相关推荐
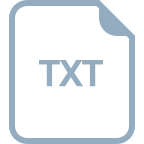
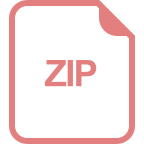
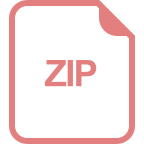
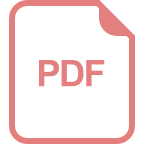
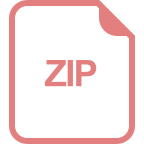
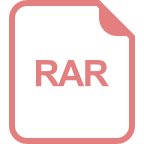
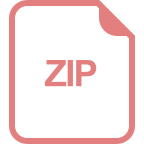
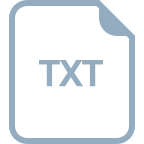
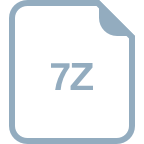
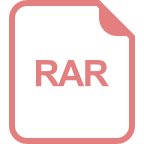
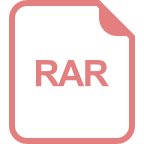
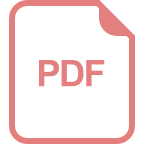
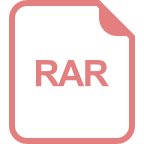
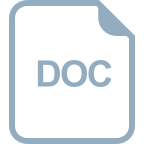
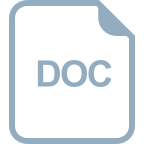

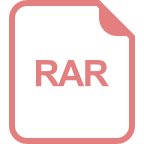