vue中使用echarts制作一个热力图
时间: 2023-11-25 10:06:40 浏览: 97
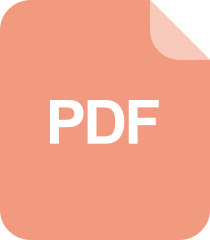
在Vue中使用echarts的方法

1. 安装echarts
```
npm install echarts --save
```
2. 引入echarts
在需要用到echarts的组件中引入echarts
```javascript
import echarts from 'echarts'
```
3. 创建一个div容器
在需要展示热力图的组件中创建一个div容器,并设置好宽高
```html
<div id="heatmap" style="width: 100%; height: 500px;"></div>
```
4. 初始化echarts
在组件的mounted生命周期函数中,初始化echarts,并传入div容器的id
```javascript
mounted() {
this.initHeatMap()
},
methods: {
initHeatMap() {
const myChart = echarts.init(document.getElementById('heatmap'))
// ...
}
}
```
5. 设置热力图的数据
```javascript
const data = [
[0, 0, 10],
[0, 1, 20],
[0, 2, 30],
[1, 0, 40],
[1, 1, 50],
[1, 2, 60],
[2, 0, 70],
[2, 1, 80],
[2, 2, 90]
]
const option = {
tooltip: {},
visualMap: {
min: 0,
max: 100,
calculable: true,
orient: 'horizontal',
left: 'center',
bottom: '15%'
},
series: [{
type: 'heatmap',
data: data
}]
}
myChart.setOption(option)
```
6. 完整代码
```javascript
<template>
<div id="heatmap" style="width: 100%; height: 500px;"></div>
</template>
<script>
import echarts from 'echarts'
export default {
mounted() {
this.initHeatMap()
},
methods: {
initHeatMap() {
const myChart = echarts.init(document.getElementById('heatmap'))
const data = [
[0, 0, 10],
[0, 1, 20],
[0, 2, 30],
[1, 0, 40],
[1, 1, 50],
[1, 2, 60],
[2, 0, 70],
[2, 1, 80],
[2, 2, 90]
]
const option = {
tooltip: {},
visualMap: {
min: 0,
max: 100,
calculable: true,
orient: 'horizontal',
left: 'center',
bottom: '15%'
},
series: [{
type: 'heatmap',
data: data
}]
}
myChart.setOption(option)
}
}
}
</script>
```
阅读全文
相关推荐
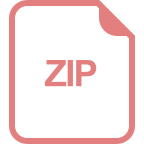
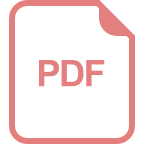
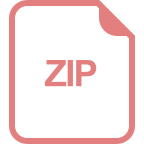
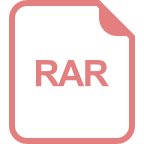
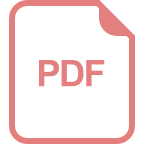
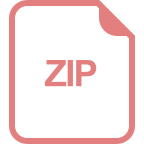
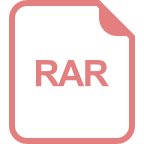
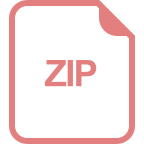
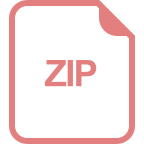
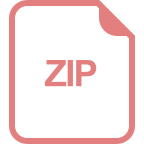
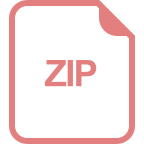
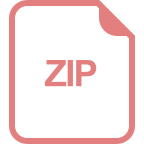
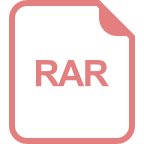
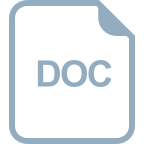
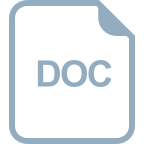
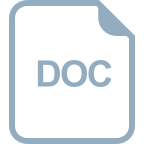
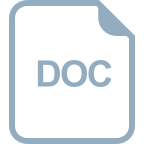
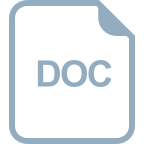