vue循环渲染一个组件多次
时间: 2023-10-29 14:26:03 浏览: 118
在Vue中,你可以使用`v-for`指令来循环渲染一个组件多次。
例如,如果你想要渲染多个`MyComponent`组件,你可以这样做:
```html
<template>
<div>
<my-component v-for="i in 5" :key="i" />
</div>
</template>
```
在上面的例子中,我们使用`v-for`指令来循环渲染`MyComponent`组件5次,用`:key`指令来指定每个组件的唯一标识符。
你也可以在循环中使用`v-bind`指令来动态传递数据给每个组件:
```html
<template>
<div>
<my-component v-for="(item, index) in items" :key="index" :data="item" />
</div>
</template>
```
在上面的例子中,我们循环渲染`MyComponent`组件,每个组件都会被传递一个`data`属性,这个属性的值来自于`items`数组中的每个元素。`:key`指令仍然用于指定每个组件的唯一标识符。
相关问题
vue3父组件循环子组件
### 回答1:
可以使用 `v-for` 指令来循环渲染子组件。在父组件模板中,使用 `v-for` 指令迭代一个数组,并使用子组件的标签名来渲染子组件。
例如,假设我们有一个名为 `ChildComponent` 的子组件,我们可以在父组件模板中使用以下代码来循环渲染多个子组件:
```html
<template>
<div>
<child-component v-for="item in items" :key="item.id" :data="item"></child-component>
</div>
</template>
```
在上面的代码中,我们使用 `v-for` 指令迭代 `items` 数组并将其绑定到子组件的 `data` 属性中。`key` 属性是必需的,以确保每个子组件都有一个唯一的标识符,以便 Vue 可以正确地更新它们。
在子组件中,我们可以使用 `props` 来接收从父组件传递的数据。例如:
```html
<template>
<div>
<p>{{ data.name }}</p>
<p>{{ data.age }}</p>
</div>
</template>
<script>
export default {
props: {
data: {
type: Object,
required: true
}
}
}
</script>
```
在上面的代码中,我们定义了一个名为 `data` 的 prop,类型为对象,并将其标记为必需的。在子组件模板中,我们可以使用 `data` 对象中的属性来显示数据。
### 回答2:
Vue3中,我们可以使用v-for指令在父组件中循环创建子组件。
首先,我们需要在父组件中声明一个数据对象,用于存储子组件的数据。例如,我们可以在父组件中定义一个数组`childComponents`来保存子组件的数据。
然后,在父组件的模板中,使用`v-for`指令来循环创建子组件。指令的形式为`v-for="item in childComponents"`,其中`item`是一个临时的变量名,代表循环过程中的每个子组件。
接下来,我们需要在循环中,使用`<child-component />`来创建子组件。例如,如果我们有一个名为`ChildComponent`的子组件,我们可以在循环中使用`<child-component :data="item" />`来创建子组件,并将循环中的每个子组件的数据传递给子组件的`data`属性。
最后,我们需要在父组件中的data选项中初始化`childComponents`数组,用于存储需要循环创建的子组件的数据。例如,我们可以将`childComponents`初始化为一个包含多个子组件数据的数组。
总之,Vue3中父组件循环子组件的步骤为:
1. 在父组件中声明一个数据对象来存储子组件的数据。
2. 在父组件模板中使用`v-for`指令循环创建子组件。
3. 在循环中使用`<child-component />`来创建子组件,并传递子组件的数据。
4. 在父组件的data选项中初始化子组件的数据数组。
以上就是使用Vue3进行父组件循环子组件的方法,希望对你有所帮助。
### 回答3:
在Vue 3中,父组件循环子组件的方法可以使用`v-for`指令。`v-for`指令是在父组件的模板中使用的,用于遍历一个数组或对象,并将子组件渲染多次。
首先,我们在父组件的模板中使用`v-for`指令来循环子组件。假设我们有一个名为`items`的数组,包含了子组件的数据。可以像这样循环子组件:
```
<template>
<div>
<child-component v-for="item in items" :key="item.id" :data="item"></child-component>
</div>
</template>
```
上述代码中,我们通过`v-for`指令遍历了`items`数组,并将每个数组元素赋值给`item`,然后在`child-component`标签上绑定了`:data`属性,将`item`对象作为数据传递给子组件。
接下来,我们需要在子组件中接收和使用父组件传递的数据。在子组件的模板中,我们可以通过`props`接收父组件传递的数据。例如,我们在子组件中定义一个名为`data`的`prop`,用于接收父组件传递的数据:
```
<template>
<div>
<p>{{ data }}</p>
</div>
</template>
<script>
export default {
props: ['data']
}
</script>
```
在上述代码中,子组件通过`props`属性定义了一个名为`data`的属性,用于接收父组件传递的数据。然后,在模板中使用`{{ data }}`输出接收到的数据。
综上所述,我们可以通过在父组件中使用`v-for`指令来循环子组件,并在子组件中使用`props`接收和使用父组件传递的数据。这样就实现了父组件循环子组件的功能。
vue中如何将一个组件引用多次但数据不一样
在Vue.js中,如果你想要在一个页面上引用一个组件多次并给每个实例提供不同的数据,可以利用Vue的动态组件或者v-for指令来实现。这里有两种常见的方式:
1. 动态组件:使用`<component :is="componentName" v-bind="componentProps"></component>`,其中`componentName`是一个字符串,可以根据需要切换不同的组件名,`componentProps`是一个对象,包含每个组件实例所需的特定数据。例如:
```html
<template>
<div>
<button @click="switchComponent('ComponentA')">显示A</button>
<button @click="switchComponent('ComponentB')">显示B</button>
<component :is="currentComponent" v-bind="currentData"></component>
</div>
</template>
<script>
export default {
data() {
return {
currentComponent: 'ComponentA',
currentData: { prop1: 'value1', prop2: 'value2' }, // 初始化数据
};
},
methods: {
switchComponent(name) {
this.currentComponent = name;
// 根据新组件的特性动态更改data对象
if (name === 'ComponentB') {
this.currentData = { prop1: 'newValue1', prop2: 'newValue2' };
}
},
},
};
</script>
```
2. v-for循环:如果数据列表不同,可以使用v-for遍历数据数组,然后渲染一个相同的组件,每个组件实例都会接收到对应数组项的数据。例如:
```html
<template>
<ul>
<li v-for="(item, index) in items" :key="index">
<my-component :data="item"></my-component>
</li>
</ul>
</template>
<script>
import MyComponent from './MyComponent.vue';
export default {
components: { MyComponent },
data() {
return {
items: [
{ prop1: 'value1', prop2: 'value2' },
{ prop1: 'value3', prop2: 'value4' },
],
};
},
};
</script>
```
在上面的例子中,`my-component`会被渲染两次,每次使用不同的数据`item`。
阅读全文
相关推荐
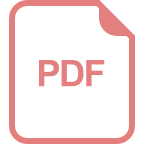
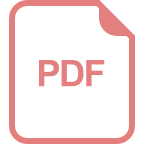
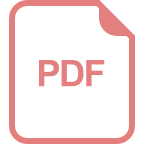
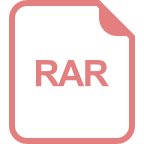
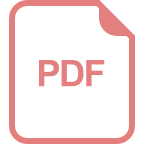
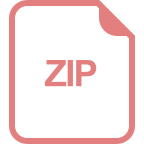
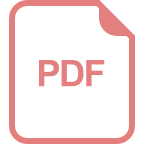
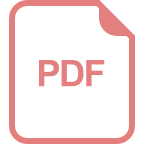
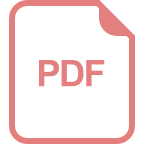
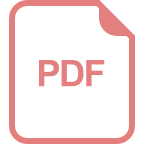
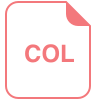
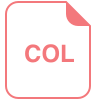
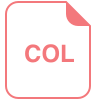

