如何把一个函数里的数组传到另外一个函数里
时间: 2024-02-27 20:55:30 浏览: 30
你可以在第一个函数中将数组作为参数传递给第二个函数,如下所示:
```python
def function1(arr):
# do something with the array
function2(arr)
def function2(arr):
# do something with the array
```
在上面的代码中,`function1` 接受一个数组 `arr` 作为参数,然后将它传递给 `function2`。在 `function2` 中,你也可以像 `function1` 中一样使用该数组。
在 Python 中,数组通常是列表(list)的形式存在,因此在函数参数中传递列表即可。如果需要传递多个数组,可以将它们放在一个元组中一起传递,如下所示:
```python
def function1(arr1, arr2):
# do something with the arrays
function2((arr1, arr2))
def function2(arrs):
arr1, arr2 = arrs
# do something with the arrays
```
在上面的代码中,`function1` 接受两个数组 `arr1` 和 `arr2` 作为参数,然后将它们放在一个元组中传递给 `function2`。在 `function2` 中,通过解包元组得到两个数组,并可以像 `function1` 中一样使用它们。
相关问题
如何把一个函数里的数组元素传到另外一个函数里
你可以在第一个函数中将数组作为参数传递给第二个函数,如下所示:
```c++
void function1(int arr[], int size) {
// do something with the array
function2(arr, size);
}
void function2(int arr[], int size) {
// do something with the array
}
```
在上面的代码中,`function1` 接受一个整数数组 `arr` 和它的大小 `size` 作为参数,然后将它们传递给 `function2`。在 `function2` 中,你也可以像 `function1` 中一样使用该数组。
将一个函数的数组元素传到下一个函数
可以使用函数参数的方式将一个函数的数组元素传到下一个函数。
例如,假设有两个函数func1和func2,func1接受一个数组作为参数,func2接受一个数组元素作为参数,可以将func1中的数组元素依次传给func2:
```
void func1(int arr[], int size) {
for (int i = 0; i < size; i++) {
func2(arr[i]);
}
}
void func2(int element) {
// do something with the element
}
```
在func1中,使用一个循环遍历数组元素,将每个元素作为参数传给func2。在func2中,可以对每个数组元素进行必要的操作。
相关推荐
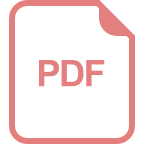
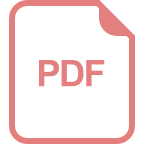
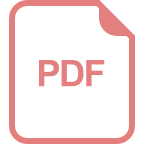












