window使用asyncio和Daphne,解决django项目耗时任务导致网页无响应
时间: 2023-11-27 18:53:13 浏览: 47
在 Django 中,通常会使用 Celery 来处理异步任务,但是如果你想要使用 asyncio 和 Daphne 来解决耗时任务导致网页无响应的问题,可以按照以下步骤进行操作:
1. 安装 daphne 和 channels:
```
pip install daphne channels
```
2. 在 Django 项目的 settings.py 中进行配置:
```
ASGI_APPLICATION = 'my_project.asgi.application'
CHANNEL_LAYERS = {
'default': {
'BACKEND': 'channels.layers.InMemoryChannelLayer',
},
}
```
3. 创建一个 asgi.py 文件,用于配置 ASGI 应用程序:
```
import os
from django.core.asgi import get_asgi_application
from channels.routing import ProtocolTypeRouter, URLRouter
from channels.auth import AuthMiddlewareStack
from my_app.routing import websocket_urlpatterns
os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'my_project.settings')
application = ProtocolTypeRouter({
"http": get_asgi_application(),
"websocket": AuthMiddlewareStack(
URLRouter(
websocket_urlpatterns
)
),
})
```
4. 创建一个 routing.py 文件,用于配置 WebSocket 路由:
```
from django.urls import re_path
from . import consumers
websocket_urlpatterns = [
re_path(r'ws/myapp/(?P<room_name>\w+)/$', consumers.MyConsumer.as_asgi()),
]
```
5. 创建一个 consumers.py 文件,用于处理 WebSocket 连接:
```
import asyncio
import json
from channels.generic.websocket import AsyncWebsocketConsumer
class MyConsumer(AsyncWebsocketConsumer):
async def connect(self):
self.room_name = self.scope['url_route']['kwargs']['room_name']
self.room_group_name = 'chat_%s' % self.room_name
await self.channel_layer.group_add(
self.room_group_name,
self.channel_name
)
await self.accept()
async def disconnect(self, close_code):
await self.channel_layer.group_discard(
self.room_group_name,
self.channel_name
)
async def receive(self, text_data):
text_data_json = json.loads(text_data)
message = text_data_json['message']
await self.channel_layer.group_send(
self.room_group_name,
{
'type': 'chat_message',
'message': message
}
)
async def chat_message(self, event):
message = event['message']
await self.send(text_data=json.dumps({
'message': message
}))
```
6. 在 views.py 中创建一个耗时任务,并使用 asyncio.run_in_executor() 方法将其运行在一个新的线程中:
```
import asyncio
from concurrent.futures import ThreadPoolExecutor
def my_view(request):
with ThreadPoolExecutor() as executor:
loop = asyncio.get_event_loop()
result = loop.run_in_executor(executor, my_long_running_task)
return HttpResponse(result)
def my_long_running_task():
# Do some heavy lifting here...
return 'Done!'
```
7. 启动 Daphne 服务器:
```
daphne my_project.asgi:application
```
现在,当用户访问包含耗时任务的页面时,它们将收到一个立即响应,而不必等待耗时任务完成。同时,你可以使用 WebSocket 来实时更新用户界面,以显示任务的进度或结果。
相关推荐
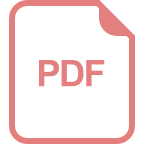
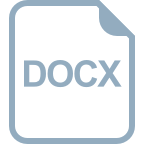
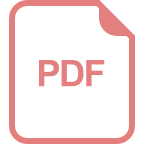














