灭火机器人的c语言代码
时间: 2023-09-03 21:13:03 浏览: 88
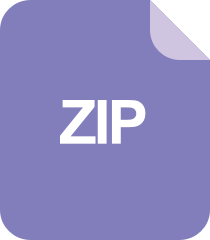
灭火机器人代码
以下是一个简单的灭火机器人的C语言代码示例,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <time.h>
#define TEMPERATURE_THRESHOLD 50 // 温度阈值,超过该温度则启动灭火程序
#define WATER_TANK_CAPACITY 100 // 水箱容量,单位为升
typedef struct {
int current_water_level; // 当前水位,初始值为满水箱
bool is_running; // 灭火机器人的运行状态,初始值为false,即未启动
} FirefightingRobot;
void detect_temperature(int current_temperature, FirefightingRobot *robot);
void start_firefighting(FirefightingRobot *robot);
void spray_water(FirefightingRobot *robot);
void stop_firefighting(FirefightingRobot *robot);
int main() {
FirefightingRobot robot = {WATER_TANK_CAPACITY, false};
// 模拟温度检测,随机生成温度值,范围为0~100
srand(time(NULL)); // 初始化随机数生成器
while (true) {
int current_temperature = rand() % 101;
detect_temperature(current_temperature, &robot);
sleep(1); // 暂停1秒钟
}
return 0;
}
void detect_temperature(int current_temperature, FirefightingRobot *robot) {
if (current_temperature > TEMPERATURE_THRESHOLD) {
start_firefighting(robot);
}
}
void start_firefighting(FirefightingRobot *robot) {
if (!robot->is_running) {
robot->is_running = true;
while (robot->current_water_level > 0) {
spray_water(robot);
sleep(1); // 暂停1秒钟
}
stop_firefighting(robot);
}
}
void spray_water(FirefightingRobot *robot) {
robot->current_water_level -= 1;
printf("喷水灭火!当前水位:%dL\n", robot->current_water_level);
}
void stop_firefighting(FirefightingRobot *robot) {
robot->is_running = false;
printf("灭火机器人停止工作!\n");
}
```
这是一个简单的灭火机器人的C语言代码,其中包含了温度检测、灭火、停止工作等函数,可以根据需要进行修改和扩展。
阅读全文
相关推荐

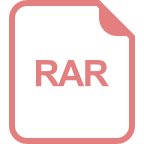
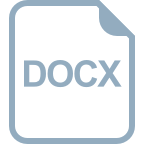
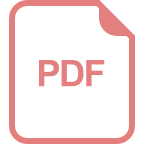
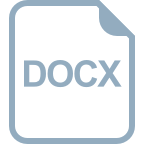
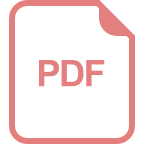
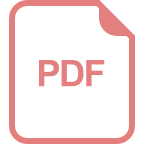
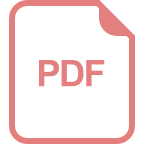
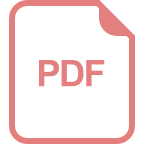
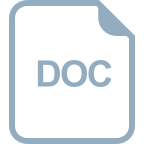
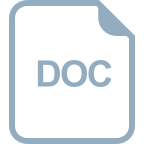
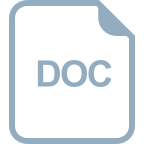
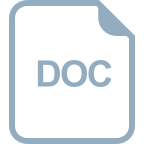
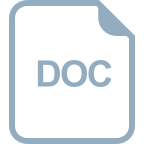