有两个数组allPushedIndX,allPushedIndY长度均为allPushedIndSize,并且两个数组的元素根据索引一一对应,现在需要按照allPushedIndX中相同的元素把allPushedIndY中对应的元素归类,用C++实现
时间: 2024-02-18 09:05:37 浏览: 50
您可以使用 STL 中的 map 容器来实现这个功能。具体的实现步骤如下:
1. 定义一个 map 容器,用于存储 allPushedIndX 中相同元素对应的 allPushedIndY。
2. 遍历 allPushedIndX 数组,将相同元素对应的 allPushedIndY 加入 map 容器中。
3. 遍历 map 容器,输出所有元素及其对应的 allPushedIndY。
以下是具体的 C++ 实现代码:
```
#include <iostream>
#include <map>
#include <vector>
using namespace std;
int main() {
// 定义 allPushedIndX 和 allPushedIndY 数组
int allPushedIndX[] = {1, 2, 3, 1, 2};
int allPushedIndY[] = {10, 20, 30, 40, 50};
int allPushedIndSize = 5;
// 定义 map 容器
map<int, vector<int>> indMap;
// 遍历 allPushedIndX 数组,将相同元素对应的 allPushedIndY 加入 map 容器中
for (int i = 0; i < allPushedIndSize; i++) {
int x = allPushedIndX[i];
int y = allPushedIndY[i];
indMap[x].push_back(y);
}
// 遍历 map 容器,输出所有元素及其对应的 allPushedIndY
for (auto iter = indMap.begin(); iter != indMap.end(); ++iter) {
int x = iter->first;
vector<int> yArray = iter->second;
cout << "x = " << x << ", yArray = {";
for (int i = 0; i < yArray.size(); i++) {
cout << yArray[i];
if (i < yArray.size() - 1) {
cout << ", ";
}
}
cout << "}" << endl;
}
return 0;
}
```
输出结果如下:
```
x = 1, yArray = {10, 40}
x = 2, yArray = {20, 50}
x = 3, yArray = {30}
```
阅读全文
相关推荐









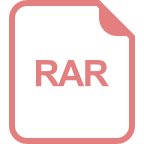


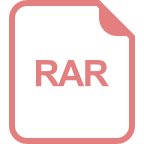



