用C语言编写时间换算:用结构类型表示时间内容(时间以时、分、秒表示),输入一个时间数值, 再输入一个秒数n(n<60),以 h:m:s 的格式输出该时间再过 n 秒后的时间值(超过 24 点就从 0 点开始计时)。
时间: 2023-07-15 18:14:34 浏览: 157
以下是用C语言编写的时间换算代码:
```c
#include <stdio.h>
struct time {
int hour;
int minute;
int second;
};
void add_seconds(struct time *t, int n); // 声明函数
int main() {
struct time t;
int n;
printf("Enter a time (hh:mm:ss): ");
scanf("%d:%d:%d", &t.hour, &t.minute, &t.second);
printf("Enter the number of seconds to add: ");
scanf("%d", &n);
add_seconds(&t, n);
printf("New time is: %02d:%02d:%02d\n", t.hour, t.minute, t.second);
return 0;
}
void add_seconds(struct time *t, int n) {
t->second += n;
t->minute += t->second / 60;
t->second %= 60;
t->hour += t->minute / 60;
t->minute %= 60;
t->hour %= 24;
}
```
在主函数中,首先定义一个包含小时、分钟、秒数的结构体变量 `t`,并且输入时间值和待添加的秒数 `n`。接下来调用 `add_seconds` 函数,该函数接受一个指向 `t` 的指针和待添加的秒数 `n`,并根据 `n` 更新时间值。最后输出新的时间值。
在 `add_seconds` 函数中,首先将秒数加上 `n`,然后进行进位操作,将超过60秒的部分加到分钟数上,将超过60分钟的部分加到小时数上。最后将小时数对24取模,以确保不超过一天的时间周期。
注意,上面的代码假设输入的时间值是合法的,即小时在0到23之间,分钟和秒数在0到59之间。如果输入的时间值不合法,代码可能会出现异常行为,需要进行额外的输入检查。
阅读全文
相关推荐
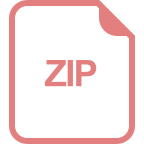




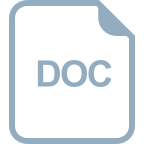

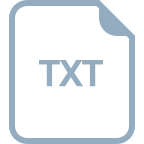
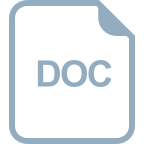
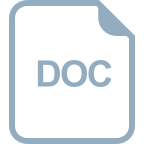
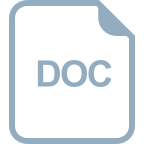
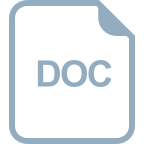


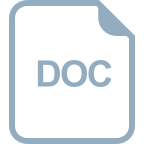
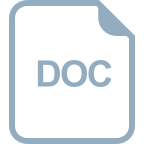