蚁群算法精英蚂蚁python
时间: 2023-11-07 22:04:33 浏览: 53
精英蚂蚁系统是蚁群算法的一种改进方法,其主要思想是引入了精英蚂蚁,以保留并传递历史最优解,从而提高算法的收敛性和搜索效果。在精英蚂蚁系统中,每一代蚂蚁群体中的蚂蚁会选择历史上找到的最好路径进行引导,并在搜索过程中更新信息素。这种方法有效地提高了算法的性能,使其更好地适应复杂的问题。
以下是精英蚂蚁系统的Python实现的简要示例:
```python
import random
class Ant:
def __init__(self, city_num):
self.city_num = city_num
self.visited = [False] * city_num
self.path = [0] * city_num
self.path[0] = random.randint(0, city_num-1)
self.visited[self.path[0]] = True
def choose_next_city(self, pheromone, alpha, beta):
current_city = self.path[-1]
probabilities = []
for next_city in range(self.city_num):
if not self.visited[next_city]:
pheromone_amount = pheromone[current_city][next_city]
heuristic_value = 1.0 / distance[current_city][next_city]
probabilities.append((next_city, pheromone_amount**alpha * heuristic_value**beta))
total = sum(prob for _, prob in probabilities)
probabilities = [(next_city, prob / total) for next_city, prob in probabilities]
probabilities.sort(key=lambda x: x[1], reverse=True)
rand = random.random()
cumulative_prob = 0
for next_city, prob in probabilities:
cumulative_prob += prob
if cumulative_prob >= rand:
return next_city
return probabilities[-1][0]
def update_path(self, next_city):
self.visited[next_city] = True
self.path.append(next_city)
def cal_path_length(self):
length = 0
for i in range(self.city_num - 1):
length += distance[self.path[i]][self.path[i+1]]
return length
def ant_colony_optimization(city_num, ant_num, iterations):
pheromone = [[1.0] * city_num for _ in range(city_num)]
best_path = None
best_length = float('inf')
for _ in range(iterations):
ants = [Ant(city_num) for _ in range(ant_num)]
for ant in ants:
for _ in range(city_num - 1):
next_city = ant.choose_next_city(pheromone, alpha, beta)
ant.update_path(next_city)
length = ant.cal_path_length()
if length < best_length:
best_length = length
best_path = ant.path
for i in range(city_num):
for j in range(city_num):
pheromone[i][j] *= evaporation_rate
for ant in ants:
for i in range(city_num - 1):
current_city, next_city = ant.path[i], ant.path[i+1]
pheromone[current_city][next_city] += deposit_rate / ant.cal_path_length()
return best_path, best_length
# 示例数据
distance = [
[0, 2, 9, 10],
[1, 0, 6, 4],
[15, 7, 0, 8],
[6, 3, 12, 0]
]
city_num = 4
ant_num = 10
iterations = 100
alpha = 1
beta = 2
evaporation_rate = 0.5
deposit_rate = 1.0
best_path, best_length = ant_colony_optimization(city_num, ant_num, iterations)
print("最佳路径:", best_path)
print("最佳路径长度:", best_length)
```
相关推荐
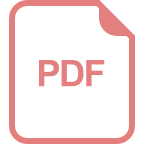

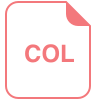












