STM32F407库函数通过软件模拟IO口的IIC通信如何实现读取INA260寄存器内的ID号实例代码
时间: 2023-07-16 11:15:06 浏览: 107
以下是使用STM32F407库函数通过软件模拟IO口的IIC通信读取INA260寄存器内的ID号的示例代码,其中使用了PA8作为SCL时钟线、PA9作为SDA数据线:
```c
#include "stm32f4xx.h"
#include "delay.h"
#define SCL_H GPIOA->BSRRL |= GPIO_Pin_8
#define SCL_L GPIOA->BSRRH |= GPIO_Pin_8
#define SDA_H GPIOA->BSRRL |= GPIO_Pin_9
#define SDA_L GPIOA->BSRRH |= GPIO_Pin_9
#define SDA_read GPIOA->IDR & GPIO_Pin_9
#define ADDR 0x80
void IIC_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_8 | GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStructure.GPIO_OType = GPIO_OType_OD;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
SCL_H;
SDA_H;
}
void IIC_Start(void)
{
SDA_H;
SCL_H;
delay_us(5);
SDA_L;
delay_us(5);
SCL_L;
}
void IIC_Stop(void)
{
SDA_L;
SCL_H;
delay_us(5);
SDA_H;
delay_us(5);
}
void IIC_Ack(void)
{
SDA_L;
SCL_H;
delay_us(5);
SCL_L;
SDA_H;
}
void IIC_NoAck(void)
{
SDA_H;
SCL_H;
delay_us(5);
SCL_L;
}
uint8_t IIC_ReadByte(void)
{
uint8_t i, dat = 0;
SDA_H;
for(i = 0; i < 8; i++)
{
dat <<= 1;
SCL_H;
delay_us(5);
if(SDA_read) dat++;
SCL_L;
delay_us(5);
}
return dat;
}
void IIC_SendByte(uint8_t dat)
{
uint8_t i;
for(i = 0; i < 8; i++)
{
if(dat & 0x80) SDA_H;
else SDA_L;
dat <<= 1;
SCL_H;
delay_us(5);
SCL_L;
delay_us(5);
}
}
uint16_t INA260_ReadReg(uint8_t regAddr)
{
uint16_t regVal = 0;
IIC_Start();
IIC_SendByte(ADDR);
if(IIC_ReadByte() == 0)
{
IIC_SendByte(regAddr);
if(IIC_ReadByte() == 0)
{
IIC_Start();
IIC_SendByte(ADDR | 0x01);
if(IIC_ReadByte() == 0)
{
regVal = (uint16_t)IIC_ReadByte() << 8;
regVal |= IIC_ReadByte();
IIC_NoAck();
}
}
}
IIC_Stop();
return regVal;
}
int main(void)
{
uint16_t idRegVal;
IIC_Init();
idRegVal = INA260_ReadReg(0xFE);
if(idRegVal == 0x227E)
{
// ID号读取成功
}
while(1);
}
```
在上述代码中,`IIC_Init()`函数用于初始化IIC通信所需的PA8和PA9引脚;`IIC_Start()`和`IIC_Stop()`函数用于IIC通信的起始和停止;`IIC_Ack()`和`IIC_NoAck()`函数用于发送和接收应答信号;`IIC_ReadByte()`函数用于读取一个字节的数据;`IIC_SendByte()`函数用于发送一个字节的数据;`INA260_ReadReg()`函数用于读取INA260中指定寄存器的值。在`main()`函数中,调用`INA260_ReadReg()`函数读取0xFE寄存器的值,并判断是否与预期的ID号0x227E相等。
阅读全文
相关推荐
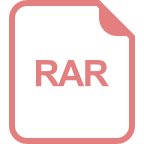
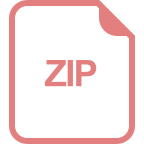
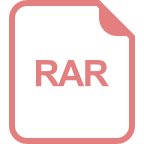
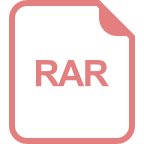
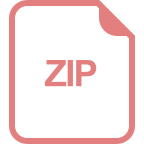
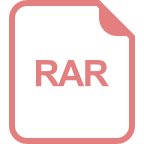
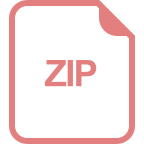
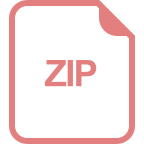
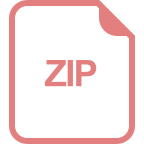
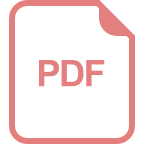
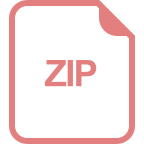
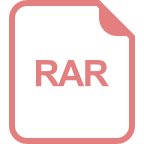
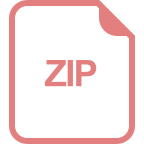
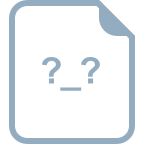
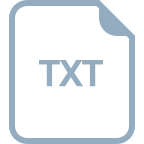