C++使用vector容器存储类的数据到txt文件中,并判断原来有没有tex文件,如果没有就创建,如果有txt文件就添加数据到末尾,最后还要从txt文件中读取数据
时间: 2023-12-14 09:36:28 浏览: 164
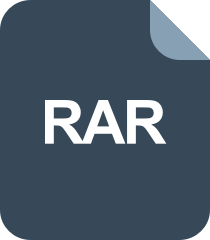
vc编程实现数据保存到txt文件里,和从txt文件里读取数据

下面是一个实现该功能的C++代码示例:
```c++
#include <iostream>
#include <fstream>
#include <vector>
using namespace std;
class Person {
public:
string name;
int age;
double height;
Person(string n, int a, double h) {
name = n;
age = a;
height = h;
}
};
int main() {
vector<Person> persons;
persons.push_back(Person("John", 25, 175.0));
persons.push_back(Person("Mary", 30, 165.5));
persons.push_back(Person("Tom", 20, 180.3));
ofstream ofs;
ofs.open("persons.txt", ios::out | ios::app);
if (ofs.is_open()) {
for (auto p : persons) {
ofs << p.name << "," << p.age << "," << p.height << endl;
}
ofs.close();
} else {
cout << "Failed to open file for writing." << endl;
return 1;
}
ifstream ifs;
ifs.open("persons.txt", ios::in);
if (ifs.is_open()) {
string line;
while (getline(ifs, line)) {
string name;
int age;
double height;
sscanf(line.c_str(), "%s,%d,%lf", &name, &age, &height);
cout << name << " " << age << " " << height << endl;
}
ifs.close();
} else {
cout << "Failed to open file for reading." << endl;
return 1;
}
return 0;
}
```
代码中,我们先定义了一个`Person`类,用于存储人的基本信息。然后创建了一个`vector`容器,存储了三个`Person`对象。接着,我们使用`ofstream`类打开`persons.txt`文件,并以追加模式写入每个`Person`对象的信息。如果文件打开成功,就循环写入并关闭文件;否则输出错误信息并返回1。
接下来,我们使用`ifstream`类打开`persons.txt`文件,并以读取模式从文件中读取每行数据,然后使用`sscanf`函数从每行数据中提取出`Person`对象的各个属性,并将其输出到控制台上。
最后,我们在`main`函数的结尾返回0表示程序正常结束。
阅读全文
相关推荐
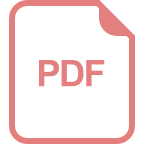
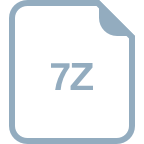
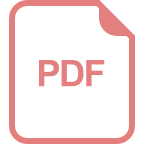
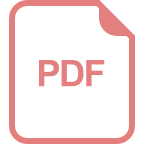
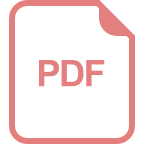
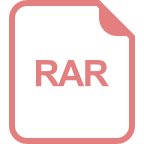
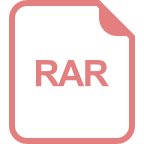
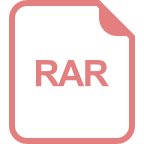
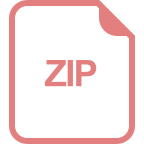
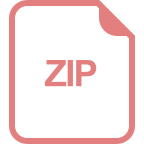
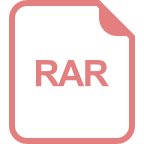
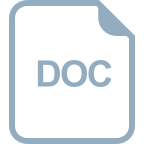