C#实现sm4/ecb/pkcs7解密
时间: 2024-10-17 18:11:08 浏览: 92
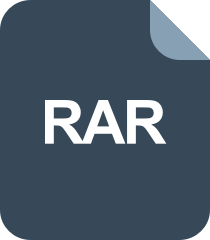
对接java AES/ECB/PKCS7Padding加密
在C#中,SM4(Secure Hashing Standard,中国加密标准算法)通常用于对数据进行加解密操作,而不是直接用于ECB模式的AES(Advanced Encryption Standard)解密。不过,如果你确实想使用ECB模式对AES进行解密,并且使用PKCS7填充(Padding),可以按照以下步骤实现:
首先,你需要引入`System.Security.Cryptography`命名空间,然后创建一个`Aes`对象并设置其ECB模式:
```csharp
using System.Security.Cryptography;
...
byte[] key = Encoding.UTF8.GetBytes("your_secret_key"); // 替换为你实际的密钥
byte[] iv = new byte[16]; // SM4一般使用16字节的IV,AES也是如此
Aes aes = Aes.Create();
aes.Key = key;
aes.IV = iv;
Mode mode = Mode.ECB; // 使用ECB模式
```
接下来,你需要创建一个`CryptoStream`来解密:
```csharp
ICryptoTransform decryptor = aes.CreateDecryptor(aes.Key, aes.IV);
using (MemoryStream msIn = new MemoryStream(Encoding.UTF8.GetBytes(base64DecryptedData))) // 替换为你的Base64解码后的输入流
using (CryptoStream csDecrypt = new CryptoStream(msIn, decryptor, CryptoStreamMode.Read))
{
using (MemoryStream msOut = new MemoryStream())
{
csDecrypt.CopyTo(msOut); // 解密
byte[] decryptedData = msOut.ToArray();
}
}
```
这里假设`base64DecryptedData`是已经解码过的Base64表示的AES加密后的数据。
注意,在实际应用中,ECB模式由于安全性较低,通常不建议单独使用。PKCS7填充也是为了弥补块密码在固定长度下不足的问题,但在某些场景中,如固定的输入大小,它仍然是可用的。
阅读全文
相关推荐
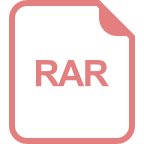












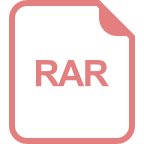


