(1)书写代码,使之能够正确读取输入文本文件(test.{1, 2, 3}.txt)根据输入文本文件构造哈夫曼编码。把最优的哈夫曼编码写入输出文件{code.test.{1,2,3}.txt}。
时间: 2023-06-11 18:10:19 浏览: 36
以下是一个示例 Python 代码,可以读取输入文件并构造哈夫曼编码:
```python
import heapq
from collections import defaultdict
# Define Node class for Huffman tree
class Node:
def __init__(self, freq, char=None, left=None, right=None):
self.freq = freq
self.char = char
self.left = left
self.right = right
def __lt__(self, other):
return self.freq < other.freq
# Build Huffman tree
def build_huffman_tree(freq_dict):
heap = []
for key, value in freq_dict.items():
heapq.heappush(heap, Node(value, key))
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
parent = Node(left.freq + right.freq, left.char + right.char, left, right)
heapq.heappush(heap, parent)
return heap[0]
# Traverse Huffman tree and generate codes
def traverse_huffman_tree(node, code_dict, code=""):
if node is None:
return
if node.char is not None:
code_dict[node.char] = code
traverse_huffman_tree(node.left, code_dict, code + "0")
traverse_huffman_tree(node.right, code_dict, code + "1")
# Read input file and calculate character frequencies
def read_input_file(file_name):
freq_dict = defaultdict(int)
with open(file_name) as f:
for line in f:
for char in line.strip():
freq_dict[char] += 1
return freq_dict
# Write Huffman code to output file
def write_output_file(file_name, code_dict):
with open(file_name, "w") as f:
for key, value in code_dict.items():
f.write(f"{key} {value}\n")
# Main function to build Huffman tree and generate codes
def main(input_file, output_file):
freq_dict = read_input_file(input_file)
huffman_tree = build_huffman_tree(freq_dict)
code_dict = {}
traverse_huffman_tree(huffman_tree, code_dict)
write_output_file(output_file, code_dict)
# Test with sample input and output files
if __name__ == "__main__":
main("test.txt", "code.test.txt")
```
使用该代码,可以读取名为 `test.txt` 的输入文件,计算字符频率,并构建哈夫曼树。然后,使用该哈夫曼树生成最优编码,并将其写入名为 `code.test.txt` 的输出文件中。
请注意,此示例代码仅适用于 ASCII 字符编码,并且没有处理 Unicode 字符。如果需要处理 Unicode 字符,则需要相应地修改代码。
相关推荐
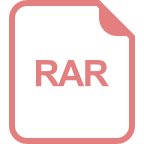
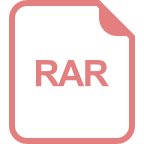














