.net Core 链接SqlServer数据库步骤
时间: 2024-01-02 17:02:56 浏览: 180
连接 SQL Server 数据库是 .NET Core 中常见的操作之一。以下是在 .NET Core 中连接 SQL Server 数据库的步骤:
1. 在 .NET Core 项目中添加 NuGet 包:Microsoft.EntityFrameworkCore.SqlServer 和 Microsoft.EntityFrameworkCore.Tools。
2. 在程序的 Startup.cs 文件中配置数据库连接字符串。在 ConfigureServices 方法中添加以下代码:
```
services.AddDbContext<YourDbContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("DefaultConnection")));
```
其中,YourDbContext 是你的 DbContext 类型,"DefaultConnection" 是你的连接字符串名称。
3. 在 appsettings.json 文件中添加连接字符串:
```
"ConnectionStrings": {
"DefaultConnection": "Server=(localdb)\\mssqllocaldb;Database=YourDatabaseName;Trusted_Connection=True;MultipleActiveResultSets=true"
}
```
其中,"Server=(localdb)\\mssqllocaldb" 是本地 SQL Server 实例名称,"YourDatabaseName" 是你的数据库名称。
4. 创建 DbContext 类。例如:
```
using Microsoft.EntityFrameworkCore;
namespace YourNamespace
{
public class YourDbContext : DbContext
{
public YourDbContext(DbContextOptions<YourDbContext> options)
: base(options)
{
}
public DbSet<YourModel> YourModels { get; set; }
}
}
```
其中,YourModel 是你的实体类,可以通过 DbSet<YourModel> 属性访问。
5. 运行以下命令创建数据库迁移:
```
dotnet ef migrations add InitialCreate
```
6. 运行以下命令将迁移应用到数据库:
```
dotnet ef database update
```
7. 现在你可以在代码中使用 DbContext 访问数据库了。例如:
```
using (var context = new YourDbContext())
{
var yourModel = new YourModel { Name = "YourName" };
context.YourModels.Add(yourModel);
context.SaveChanges();
}
```
以上是连接 SQL Server 数据库的基本步骤。当然,具体的实现可能会因为项目的不同而有所出入,但是以上步骤可以作为一个基础框架。
阅读全文
相关推荐





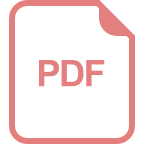
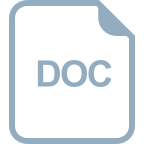
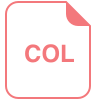
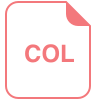
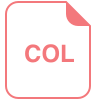
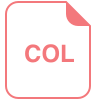
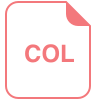
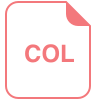
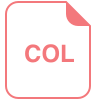
