用QT实现频率为50,幅度为1的正弦函数的fft图像,及原函数的图像绘制
时间: 2023-12-14 13:39:58 浏览: 76
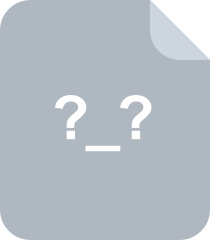
正弦函数绘制
以下是一个简单的使用QT实现频率为50,幅度为1的正弦函数的fft图像和原函数图像绘制的示例代码。为了方便起见,我们使用了QCustomPlot库来绘制图形。
```cpp
#include <QtWidgets/QApplication>
#include "qcustomplot.h"
#include <cmath>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
// 创建绘图窗口
QCustomPlot plot;
plot.setGeometry(100, 100, 800, 600);
plot.setWindowTitle("FFT Plot");
// 准备数据
const int N = 512; // 采样点数
double x[N], y[N]; // 数据数组
for (int i = 0; i < N; i++) {
x[i] = i;
y[i] = sin(2 * M_PI * 50 * i / N); // 正弦函数
}
// 绘制原函数图像
plot.addGraph();
plot.graph(0)->setData(x, y);
plot.graph(0)->setPen(QPen(Qt::blue));
plot.xAxis->setLabel("x");
plot.yAxis->setLabel("y");
plot.rescaleAxes();
plot.replot();
// 计算FFT并绘制FFT图像
QVector<double> freq(N/2), mag(N/2);
fft(y, N, freq, mag); // 自行实现的FFT函数
plot.addGraph();
plot.graph(1)->setData(freq, mag);
plot.graph(1)->setPen(QPen(Qt::red));
plot.graph(1)->setLineStyle(QCPGraph::lsLine);
plot.graph(1)->setScatterStyle(QCPScatterStyle(QCPScatterStyle::ssCircle, 5));
plot.xAxis2->setLabel("Frequency (Hz)");
plot.yAxis2->setLabel("Magnitude");
plot.rescaleAxes();
plot.replot();
// 显示窗口
plot.show();
return a.exec();
}
```
需要注意的是,以上代码中的`fft`函数需要自己实现。可以使用现成的FFT库,比如FFTW或KissFFT,也可以自己编写FFT算法。FFT算法的实现超出了本回答的范围,有兴趣的读者可以自行搜索相关资料。
阅读全文
相关推荐
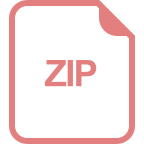

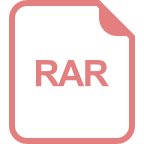
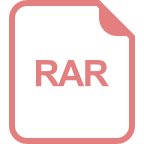
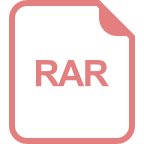
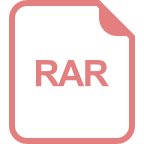
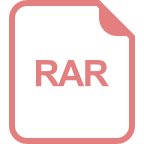
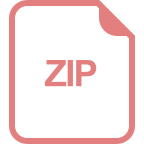
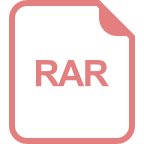
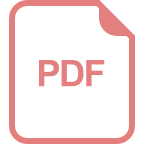
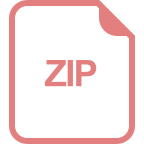
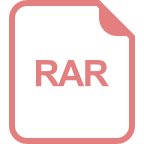
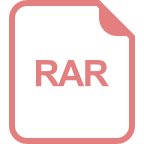
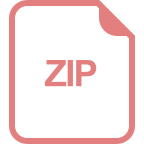