用java编写一个多线程
时间: 2023-09-15 22:05:46 浏览: 73
### 回答1:
要使用 Java 编写一个多线程程序,你可以使用以下方法之一:
1. 继承 java.lang.Thread 类:可以创建一个继承 Thread 类的新类,并重写该类的 run() 方法。然后,可以创建该类的实例,并调用它的 start() 方法来启动线程。
```
class MyThread extends Thread {
public void run() {
// your code here
}
}
// To start a thread, you create an instance of the class and call its start() method
MyThread myThread = new MyThread();
myThread.start();
```
2. 实现 java.lang.Runnable 接口:可以创建一个实现了 Runnable 接口的类,并重写该接口的 run() 方法。然后,可以创建一个 Thread 实例,并将该 Runnable 实例作为参数传递给该 Thread 实例。最后,可以调用 start() 方法启动线程。
```
class MyRunnable implements Runnable {
public void run() {
// your code here
}
}
// To start a thread, you create an instance of the Thread class and pass a Runnable instance to its constructor
MyRunnable myRunnable = new MyRunnable();
Thread myThread = new Thread(myRunnable);
myThread.start();
```
### 回答2:
使用Java编写多线程可以通过继承Thread类或实现Runnable接口来实现。
1. 继承Thread类:
```java
public class MyThread extends Thread {
public void run() {
// 线程执行的逻辑
}
}
public class Main {
public static void main(String[] args) {
MyThread thread = new MyThread();
thread.start(); // 启动线程
}
}
```
2. 实现Runnable接口:
```java
public class MyRunnable implements Runnable {
public void run() {
// 线程执行的逻辑
}
}
public class Main {
public static void main(String[] args) {
Thread thread = new Thread(new MyRunnable());
thread.start(); // 启动线程
}
}
```
在多线程中,可以同时执行多个线程,每个线程独立执行自己的任务。可以使用Thread类的start()方法启动线程,线程在运行时调用run()方法执行具体的逻辑。
多线程编程的好处是可以提高程序的效率和响应速度。通过合理地使用多线程,可以利用多核处理器并行处理任务,提升系统的整体性能。
但是多线程编程也需要注意线程安全的问题,如通过使用synchronized关键字或锁机制来保证共享资源的正确访问,避免数据竞争等问题。另外还需注意资源的释放和线程的生命周期管理,以避免内存泄漏和僵尸线程等问题。
### 回答3:
使用Java编写多线程的方法有多种,以下是一个示例:
```java
public class MultiThreadExample {
public static void main(String[] args) {
// 创建并启动线程
MyThread thread1 = new MyThread("线程1");
MyThread thread2 = new MyThread("线程2");
thread1.start();
thread2.start();
}
static class MyThread extends Thread {
private String name;
public MyThread(String name) {
this.name = name;
}
@Override
public void run() {
for (int i = 1; i <= 10; i++) {
System.out.println(name + ": " + i);
try {
Thread.sleep(1000); // 暂停1秒
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
```
上述示例中,我们创建了一个名为`MyThread`的自定义线程类,它继承自`Thread`类。在`run`方法中,我们循环输出当前线程的名称和计数器的值,并通过`Thread.sleep()`暂停1秒。在`main`方法中,我们创建了两个`MyThread`对象,并分别启动了它们。
运行该代码,输出类似于以下内容的结果:
```
线程1: 1
线程2: 1
线程2: 2
线程1: 2
线程1: 3
线程2: 3
...
```
这个例子展示了如何使用Java编写多线程。通过继承`Thread`类并重写`run`方法,我们可以创建自定义的线程逻辑。每个线程都独立运行,并且可以并发地执行不同的任务。
相关推荐
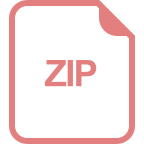
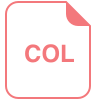
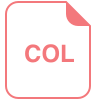
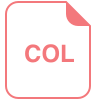

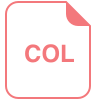









