c语言代码实现以下:开发一个字符版本的仿真软件,实现键盘控制老鼠在迷宫中找蛋糕。其中老鼠用@表示,蛋 糕用%表示,迷宫中的空间和墙壁分别用英文的句号.和#表示。为了让老鼠可以在迷宫中移动,我们通过让用户输入特定的移动指令来实现。用4个大写或者小写的字母来表示不同的移动方向:W上,A左,S下,D右。如果传入的是其它字符,函数会打印出:"WARNING:Didn’trecognize move command”,(并返回空值NULL)
时间: 2024-03-17 08:44:55 浏览: 10
以下是实现该功能的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define ROWS 10 // 迷宫行数
#define COLS 10 // 迷宫列数
// 迷宫地图
char maze[ROWS][COLS] = {
{'#', '#', '#', '#', '#', '#', '#', '#', '#', '#'},
{'#', '.', '.', '.', '#', '.', '.', '.', '.', '#'},
{'#', '.', '#', '.', '#', '.', '#', '#', '.', '#'},
{'#', '.', '#', '.', '.', '.', '.', '.', '.', '#'},
{'#', '.', '#', '#', '#', '.', '#', '#', '.', '#'},
{'#', '.', '.', '.', '#', '.', '.', '.', '.', '#'},
{'#', '#', '#', '.', '#', '#', '#', '.', '#', '#'},
{'#', '.', '.', '.', '.', '.', '.', '.', '.', '#'},
{'#', '#', '#', '#', '#', '.', '#', '#', '.', '#'},
{'#', '.', '.', '.', '#', '.', '.', '.', '%', '#'}
};
// 老鼠位置
int mouse_row = 1;
int mouse_col = 1;
// 查找目标字符在迷宫中的位置
void find_position(char target, int* row, int* col) {
int i, j;
for (i = 0; i < ROWS; i++) {
for (j = 0; j < COLS; j++) {
if (maze[i][j] == target) {
*row = i;
*col = j;
return;
}
}
}
}
// 移动老鼠
void move(char direction) {
switch (direction) {
case 'W':
case 'w':
if (maze[mouse_row-1][mouse_col] != '#') {
maze[mouse_row][mouse_col] = '.';
mouse_row--;
}
break;
case 'A':
case 'a':
if (maze[mouse_row][mouse_col-1] != '#') {
maze[mouse_row][mouse_col] = '.';
mouse_col--;
}
break;
case 'S':
case 's':
if (maze[mouse_row+1][mouse_col] != '#') {
maze[mouse_row][mouse_col] = '.';
mouse_row++;
}
break;
case 'D':
case 'd':
if (maze[mouse_row][mouse_col+1] != '#') {
maze[mouse_row][mouse_col] = '.';
mouse_col++;
}
break;
default:
printf("WARNING: Didn't recognize move command.\n");
return;
}
maze[mouse_row][mouse_col] = '@';
}
// 打印迷宫地图
void print_maze() {
int i, j;
for (i = 0; i < ROWS; i++) {
for (j = 0; j < COLS; j++) {
printf("%c", maze[i][j]);
}
printf("\n");
}
}
int main() {
int egg_row, egg_col;
find_position('%', &egg_row, &egg_col); // 查找蛋糕位置
while (1) {
system("clear"); // 清屏
print_maze(); // 打印迷宫地图
char direction;
printf("Enter move command (WASD): ");
scanf(" %c", &direction);
move(direction); // 移动老鼠
if (mouse_row == egg_row && mouse_col == egg_col) {
printf("Congratulations! You found the cake!\n");
break;
}
}
return 0;
}
```
使用该程序可以在命令行窗口中进行迷宫寻宝游戏,玩家根据提示输入移动指令,直至找到蛋糕。
相关推荐
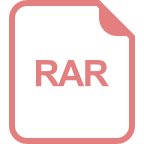
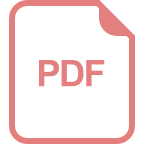
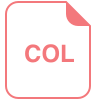
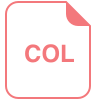
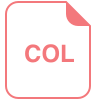
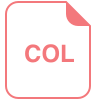
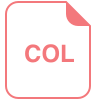









