编写一个创建文件、输入多个班级成绩、保存文件,查询成绩、修改成绩、显示所有的学生成绩,班级成绩复制到另一个文件的功能的C语言完整程序代码
时间: 2024-01-29 17:03:57 浏览: 100
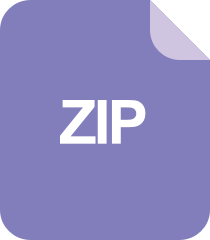
可以在文件里面存储学生信息,可以建立并删除学生成绩,可以按班级查询学生成绩.zip
好的,以下是一个简单的实现上述功能的C语言程序的代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct student {
int id;
char name[20];
float score;
};
void write_file() {
FILE *fp;
struct student stu;
int n, i;
printf("请输入学生数量:");
scanf("%d", &n);
fp = fopen("scores.txt", "w");
if (fp == NULL) {
printf("文件打开失败!\n");
exit(1);
}
for (i = 0; i < n; i++) {
printf("请输入第%d个学生的学号、姓名、成绩:", i+1);
scanf("%d %s %f", &stu.id, stu.name, &stu.score);
fprintf(fp, "%d %s %.2f\n", stu.id, stu.name, stu.score);
}
fclose(fp);
}
void read_file() {
FILE *fp;
struct student stu;
int id, choice;
float score;
char name[20];
fp = fopen("scores.txt", "r");
if (fp == NULL) {
printf("文件打开失败!\n");
exit(1);
}
printf("请输入要查询的学生的学号:");
scanf("%d", &id);
while (fscanf(fp, "%d %s %f", &stu.id, stu.name, &stu.score) != EOF) {
if (stu.id == id) {
printf("该学生信息如下:\n");
printf("学号:%d,姓名:%s,成绩:%.2f\n", stu.id, stu.name, stu.score);
printf("请选择要执行的操作:\n");
printf("1. 修改成绩\n");
printf("2. 返回\n");
scanf("%d", &choice);
switch (choice) {
case 1:
printf("请输入新的成绩:");
scanf("%f", &score);
stu.score = score;
break;
case 2:
break;
default:
printf("无效的选项!\n");
break;
}
if (fseek(fp, -(long)sizeof(struct student), SEEK_CUR) != 0) {
printf("文件指针定位失败!\n");
exit(1);
}
fprintf(fp, "%d %s %.2f\n", stu.id, stu.name, stu.score);
printf("成绩修改成功!\n");
break;
}
}
if (feof(fp)) {
printf("未找到该学生信息!\n");
}
fclose(fp);
}
void display_all() {
FILE *fp;
struct student stu;
fp = fopen("scores.txt", "r");
if (fp == NULL) {
printf("文件打开失败!\n");
exit(1);
}
printf("学号\t姓名\t成绩\n");
while (fscanf(fp, "%d %s %f", &stu.id, stu.name, &stu.score) != EOF) {
printf("%d\t%s\t%.2f\n", stu.id, stu.name, stu.score);
}
fclose(fp);
}
void copy_file() {
FILE *fp1, *fp2;
struct student stu;
fp1 = fopen("scores.txt", "r");
if (fp1 == NULL) {
printf("源文件打开失败!\n");
exit(1);
}
fp2 = fopen("scores_copy.txt", "w");
if (fp2 == NULL) {
printf("目标文件打开失败!\n");
exit(1);
}
while (fscanf(fp1, "%d %s %f", &stu.id, stu.name, &stu.score) != EOF) {
fprintf(fp2, "%d %s %.2f\n", stu.id, stu.name, stu.score);
}
printf("文件复制成功!\n");
fclose(fp1);
fclose(fp2);
}
int main() {
int choice;
while (1) {
printf("\n请选择要执行的操作:\n");
printf("1. 创建文件并输入多个班级成绩\n");
printf("2. 查询成绩并修改\n");
printf("3. 显示所有学生成绩\n");
printf("4. 将班级成绩复制到另一个文件\n");
printf("5. 退出程序\n");
scanf("%d", &choice);
switch (choice) {
case 1:
write_file();
break;
case 2:
read_file();
break;
case 3:
display_all();
break;
case 4:
copy_file();
break;
case 5:
exit(0);
default:
printf("无效的选项!\n");
break;
}
}
return 0;
}
```
这个程序中,我们使用了结构体来存储学生信息,用文件来存储多个学生的成绩。程序提供了四个功能,分别是创建文件并输入多个班级成绩、查询成绩并修改、显示所有学生成绩、将班级成绩复制到另一个文件。用户可以通过选择菜单中的选项来执行不同的功能。
阅读全文
相关推荐
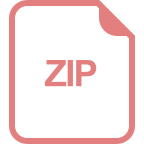



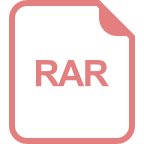
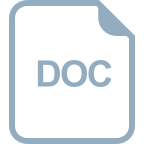
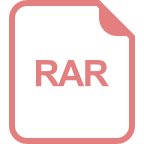
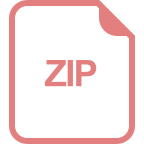
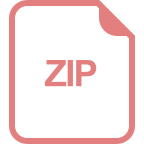
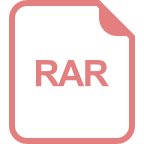
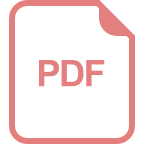
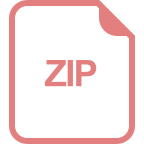
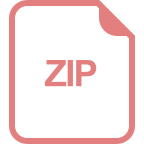
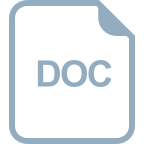
