帮我写一下springboot+JS 数据传输加密的代码,要带注释
时间: 2024-10-18 15:20:24 浏览: 3
Spring Boot应用中,我们常常需要对数据传输进行加密保护,尤其是涉及到敏感信息的时候。一个常用的加密技术是HTTPS,它通过SSL/TLS协议提供数据传输的安全性。下面是一个简单的示例,展示了如何配置Spring Boot应用使用HTTPS并启用JWT(JSON Web Tokens)进行身份验证:
```java
// 引入必要的依赖
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.http.converter.json.Jackson2ObjectMapperBuilderCustomizer;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.oauth2.core.jwt.Jwt;
@SpringBootApplication
public class App {
// 启动应用
public static void main(String[] args) {
SpringApplication.run(App.class, args);
}
// 配置JWT加密
@Configuration
public class JwtConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
// 使用HTTPS,开启安全请求处理
http.requiresChannel()
.anyRequest().requiresSecure()
.and()
.csrf().disable();
// 自定义JWT处理器
http.authorizeRequests()
.antMatchers("/api/**").authenticated()
.and()
.oauth2ResourceServer().jwt();
}
// 自定义JWT编码解码
@Bean
public JwtDecoder jwtDecoder() {
return new Jwts().setSigningKey("your-secret-key") // 替换为你自己的密钥
.setJwtIdGenerator(Jwts::createJwtId); // JWT ID生成
}
// 这里只是一个简化的例子,实际项目可能还需要配合TokenService和认证中间件
// 更详细的JWT处理可能涉及序列化/反序列化、刷新令牌等功能
}
}
```
在这个例子中,`configure()`方法设置了HTTPS安全通道,并关闭了CSRF保护。`jwtDecoder()`方法用于创建JWT解码器,这里的`"your-secret-key"`应该替换为你的私有密钥。
阅读全文
相关推荐
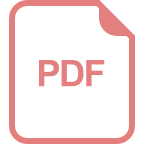
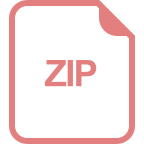
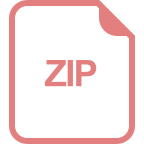















