python怎么剪切文件夹
时间: 2023-08-31 14:12:38 浏览: 94
你可以使用 `shutil` 模块来剪切文件夹。具体操作如下:
```python
import shutil
# 将 /path/to/folder 剪切到 /new/path/to/folder
shutil.move('/path/to/folder', '/new/path/to/folder')
```
其中,`shutil.move(src, dst)` 函数用于将 `src` 文件或文件夹移动到 `dst` 目录下。如果 `dst` 不存在,则会被创建。如果 `src` 和 `dst` 在同一文件系统中,则会直接移动,否则会先复制再删除原文件。
注意,在进行操作前请确保有足够的权限,以免出现不必要的错误。
相关问题
python批量剪切文件到指定文件夹
您可以使用Python的shutil模块来批量剪切文件到指定文件夹。下面是一个示例代码:
```python
import os
import shutil
# 源文件夹路径
source_folder = '/path/to/source/folder/'
# 目标文件夹路径
destination_folder = '/path/to/destination/folder/'
# 遍历源文件夹中的所有文件
for filename in os.listdir(source_folder):
# 构建源文件路径和目标文件路径
source_file = os.path.join(source_folder, filename)
destination_file = os.path.join(destination_folder, filename)
# 如果文件是文件夹,则跳过
if os.path.isdir(source_file):
continue
# 剪切文件到目标文件夹
shutil.move(source_file, destination_file)
print('所有文件已成功剪切到指定文件夹!')
```
在上面的代码中,您需要将`source_folder`和`destination_folder`替换为您实际使用的文件夹路径。代码会遍历源文件夹中的所有文件,并将它们剪切到目标文件夹中。如果源文件夹中有子文件夹,它们将被忽略。
python筛选剪切出一个文件夹里空的txt文本文件
在Python中,你可以使用os模块来操作文件和目录,结合shutil库来删除空文件。以下是一个简单的步骤来筛选并剪切(实际这里是删除)文件夹中所有空的.txt文本文件:
```python
import os
import shutil
def filter_empty_txt_files(directory):
# 遍历指定目录及其所有子目录
for root, dirs, files in os.walk(directory):
# 对于每个.txt文件
for file in files:
if file.endswith('.txt'):
# 检查文件大小是否为0,如果是,则它为空
if os.path.getsize(os.path.join(root, file)) == 0:
# 删除(或打印出如果只想检查而不想真的删除)
print(f"Found empty file: {os.path.join(root, file)}")
# 如果你想删除空文件
# shutil.rmtree(os.path.join(root, file), ignore_errors=True)
# 调用函数,并传入你想要检查的文件夹路径
filter_empty_txt_files('/path/to/your/directory')
```
请注意,`shutil.rmtree`会递归地删除整个文件夹,所以在删除前最好确认文件夹中没有其他重要数据。
阅读全文
相关推荐
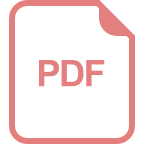
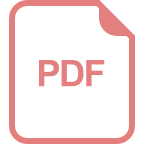
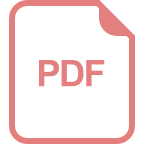
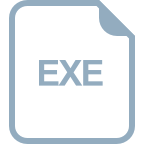










