vue3 vant buttom 绑定点击事件
时间: 2023-06-07 22:10:52 浏览: 392
好的,关于你的问题,绑定点击事件可以使用 v-on 或 @ 符号。示例代码如下:
```
<template>
<van-button @click="handleClick">Click me!</van-button>
</template>
<script>
import { defineComponent } from 'vue';
import { Button } from 'vant';
export default defineComponent({
components: {
[Button.name]: Button,
},
methods: {
handleClick() {
console.log('Button clicked!');
},
},
});
</script>
```
在模板中,我们使用 vant 的 Button 组件,并使用 @click 绑定了 handleClick 方法。在脚本中,我们需要首先导入 Button 组件并注册为本地组件,然后定义 handleClick 方法来处理按钮点击事件。当用户点击按钮时,会触发 handleClick 方法,从而打印出一条提示信息。
希望这个答案能够解决你的问题!
相关问题
vue3动态绑定点击事件
在Vue 3中,你可以使用`v-on`指令来动态绑定点击事件。下面是一个示例:
```html
<template>
<button v-on:click="handleClick">点击我</button>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
// 定义一个响应式的变量
const count = ref(0);
// 定义点击事件的处理函数
const handleClick = () => {
count.value++;
};
return {
handleClick
};
}
};
</script>
```
在上面的示例中,我们使用`v-on:click`指令将`handleClick`方法绑定到按钮的点击事件上。通过点击按钮,`count`变量的值会加1。 这里还使用了Vue 3中的`ref`函数来创建一个响应式的变量`count`。
vue3给button绑定键盘事件
在 Vue3 中,你可以使用 `@keydown` 和 `@keyup` 指令来绑定键盘事件。下面是一个示例,为按钮绑定 `Enter` 键的按下事件:
```html
<template>
<button @keydown.enter="onEnterKeyDown">Click me</button>
</template>
<script>
export default {
methods: {
onEnterKeyDown() {
console.log('Enter key was pressed');
// 处理 Enter 键被按下的逻辑
}
}
}
</script>
```
在上面的示例中,我们使用 `@keydown.enter` 指令来绑定 `Enter` 键的按下事件,并在 `onEnterKeyDown` 方法中处理按下事件的逻辑。你可以根据需要修改指令和方法名,以适应其他键盘事件的绑定。
相关推荐
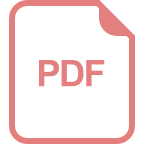
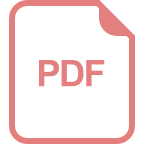
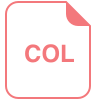
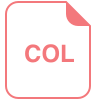
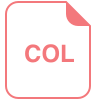
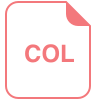
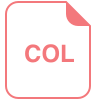







