帮我写一个java+mysql+gui界面图书管理系统
时间: 2023-07-29 19:05:47 浏览: 77
很高兴为您提供帮助。下面是一个简单的Java图书管理系统示例,使用MySQL数据库和Swing GUI界面。请注意,这只是一个简单的示例,您需要根据自己的需求进行修改和完善。
首先,您需要创建一个MySQL数据库,其中包含一个名为books的表,该表具有以下列:
```
id INT(11) AUTO_INCREMENT PRIMARY KEY
title VARCHAR(255)
author VARCHAR(255)
publisher VARCHAR(255)
year INT(11)
```
然后,您需要为系统创建一个GUI界面。以下是一个简单的示例:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class LibraryGUI implements ActionListener {
private JFrame frame;
private JLabel titleLabel, authorLabel, publisherLabel, yearLabel, idLabel;
private JTextField titleField, authorField, publisherField, yearField, idField;
private JButton addButton, deleteButton, updateButton, searchButton;
public LibraryGUI() {
frame = new JFrame("图书管理系统");
frame.setSize(400, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel(new GridLayout(6, 2));
idLabel = new JLabel("ID:");
idField = new JTextField(10);
titleLabel = new JLabel("书名:");
titleField = new JTextField(10);
authorLabel = new JLabel("作者:");
authorField = new JTextField(10);
publisherLabel = new JLabel("出版社:");
publisherField = new JTextField(10);
yearLabel = new JLabel("年份:");
yearField = new JTextField(10);
addButton = new JButton("添加");
addButton.addActionListener(this);
deleteButton = new JButton("删除");
deleteButton.addActionListener(this);
updateButton = new JButton("更新");
updateButton.addActionListener(this);
searchButton = new JButton("查询");
searchButton.addActionListener(this);
panel.add(idLabel);
panel.add(idField);
panel.add(titleLabel);
panel.add(titleField);
panel.add(authorLabel);
panel.add(authorField);
panel.add(publisherLabel);
panel.add(publisherField);
panel.add(yearLabel);
panel.add(yearField);
panel.add(addButton);
panel.add(deleteButton);
panel.add(updateButton);
panel.add(searchButton);
frame.add(panel);
frame.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
addBook();
} else if (e.getSource() == deleteButton) {
deleteBook();
} else if (e.getSource() == updateButton) {
updateBook();
} else if (e.getSource() == searchButton) {
searchBook();
}
}
private void addBook() {
// 将新书添加到数据库中
}
private void deleteBook() {
// 从数据库中删除书籍
}
private void updateBook() {
// 更新数据库中的书籍信息
}
private void searchBook() {
// 在数据库中查找书籍
}
}
```
接下来,您需要实现添加、删除、更新和搜索书籍的方法。以下是一个简单的示例:
```
import java.sql.*;
public class LibraryDAO {
private Connection connection;
public LibraryDAO() {
try {
Class.forName("com.mysql.jdbc.Driver");
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/library", "root", "password");
} catch (Exception e) {
e.printStackTrace();
}
}
public void addBook(String title, String author, String publisher, int year) {
try {
PreparedStatement statement = connection.prepareStatement("INSERT INTO books (title, author, publisher, year) VALUES (?, ?, ?, ?)");
statement.setString(1, title);
statement.setString(2, author);
statement.setString(3, publisher);
statement.setInt(4, year);
statement.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}
}
public void deleteBook(int id) {
try {
PreparedStatement statement = connection.prepareStatement("DELETE FROM books WHERE id = ?");
statement.setInt(1, id);
statement.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}
}
public void updateBook(int id, String title, String author, String publisher, int year) {
try {
PreparedStatement statement = connection.prepareStatement("UPDATE books SET title = ?, author = ?, publisher = ?, year = ? WHERE id = ?");
statement.setString(1, title);
statement.setString(2, author);
statement.setString(3, publisher);
statement.setInt(4, year);
statement.setInt(5, id);
statement.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}
}
public ResultSet searchBook(int id) {
ResultSet result = null;
try {
PreparedStatement statement = connection.prepareStatement("SELECT * FROM books WHERE id = ?");
statement.setInt(1, id);
result = statement.executeQuery();
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
}
```
最后,您需要将GUI和DAO连接起来。以下是一个简单的示例:
```
public class LibraryApp {
private static LibraryDAO dao = new LibraryDAO();
private static LibraryGUI gui = new LibraryGUI();
public static void main(String[] args) {
}
private static void addBook() {
String title = gui.getTitle();
String author = gui.getAuthor();
String publisher = gui.getPublisher();
int year = gui.getYear();
dao.addBook(title, author, publisher, year);
}
private static void deleteBook() {
int id = gui.getId();
dao.deleteBook(id);
}
private static void updateBook() {
int id = gui.getId();
String title = gui.getTitle();
String author = gui.getAuthor();
String publisher = gui.getPublisher();
int year = gui.getYear();
dao.updateBook(id, title, author, publisher, year);
}
private static void searchBook() {
int id = gui.getId();
ResultSet result = dao.searchBook(id);
// 显示结果
}
}
```
请注意,这只是一个简单的示例。您需要根据自己的需求进行修改和完善。
相关推荐
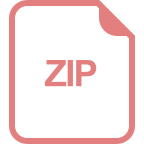














