read_image(AVFrame *, char*)
时间: 2024-01-17 15:09:40 浏览: 109
As an AI language model, I can interpret this function as a method that takes in an AVFrame pointer and a character pointer as parameters, and returns data related to an image file.
However, without knowing the context and purpose of this function, it is difficult to provide a more accurate interpretation. The AVFrame pointer might refer to a video frame, and the character pointer might contain the file path to an image that needs to be read. The function might use a library or API to read the image file and store its data in the AVFrame.
相关问题
ffempg硬件缩放C++使用scale_cuda过滤器缩放视频
ffmpeg是一个非常强大的开源多媒体处理库,它支持各种格式的视频和音频的编解码、转换、过滤等操作。在ffmpeg中,scale_cuda过滤器可以使用GPU加速进行视频缩放。
下面是使用scale_cuda过滤器进行视频缩放的C++代码示例:
```c++
#include <iostream>
#include <string>
#include <opencv2/opencv.hpp>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/opt.h>
#include <libavutil/imgutils.h>
#include <libavfilter/avfilter.h>
#include <libavfilter/buffersink.h>
#include <libavfilter/buffersrc.h>
using namespace std;
using namespace cv;
int main(int argc, char* argv[])
{
if (argc != 4)
{
cout << "Usage: ./scale_cuda <input_file> <width> <height>" << endl;
return -1;
}
string input_file = argv[1];
int dst_width = stoi(argv[2]);
int dst_height = stoi(argv[3]);
// 初始化ffmpeg
av_register_all();
avfilter_register_all();
// 打开输入文件
AVFormatContext* input_ctx = nullptr;
if (avformat_open_input(&input_ctx, input_file.c_str(), nullptr, nullptr) < 0)
{
cout << "Failed to open input file" << endl;
return -1;
}
// 获取视频流信息
if (avformat_find_stream_info(input_ctx, nullptr) < 0)
{
cout << "Failed to find stream information" << endl;
avformat_close_input(&input_ctx);
return -1;
}
// 获取视频流索引
int video_stream_index = -1;
for (unsigned int i = 0; i < input_ctx->nb_streams; i++)
{
if (input_ctx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO)
{
video_stream_index = i;
break;
}
}
if (video_stream_index == -1)
{
cout << "Failed to find video stream" << endl;
avformat_close_input(&input_ctx);
return -1;
}
// 创建输入流上下文
AVCodecParameters* codecpar = input_ctx->streams[video_stream_index]->codecpar;
AVStream* input_stream = input_ctx->streams[video_stream_index];
AVCodec* codec = avcodec_find_decoder(codecpar->codec_id);
AVCodecContext* codec_ctx = avcodec_alloc_context3(codec);
avcodec_parameters_to_context(codec_ctx, codecpar);
avcodec_open2(codec_ctx, codec, nullptr);
AVFrame* raw_frame = av_frame_alloc();
AVPacket* packet = av_packet_alloc();
// 创建输出流上下文
AVFilterContext* buffersrc_ctx = nullptr;
AVFilterContext* buffersink_ctx = nullptr;
AVFilterGraph* filter_graph = avfilter_graph_alloc();
// 创建buffersrc过滤器
AVFilter* buffersrc = avfilter_get_by_name("buffer");
AVDictionary* options = nullptr;
av_dict_set(&options, "video_size", "1920x1080", 0);
av_dict_set(&options, "pix_fmt", "0", 0);
av_dict_set(&options, "time_base", "1/25", 0);
av_dict_set(&options, "pixel_aspect", "0/1", 0);
char args[512];
snprintf(args, sizeof(args), "video_size=%dx%d:pix_fmt=%d:time_base=%d/%d:pixel_aspect=%d/%d",
codec_ctx->width, codec_ctx->height, codec_ctx->pix_fmt,
input_stream->time_base.num, input_stream->time_base.den,
codecpar->sample_aspect_ratio.num, codecpar->sample_aspect_ratio.den);
avfilter_graph_create_filter(&buffersrc_ctx, buffersrc, "in", args, options, filter_graph);
// 创建scale_cuda过滤器
AVFilter* scale_cuda = avfilter_get_by_name("scale_cuda");
snprintf(args, sizeof(args), "%d:%d", dst_width, dst_height);
avfilter_graph_create_filter(&buffersink_ctx, scale_cuda, "out", args, nullptr, filter_graph);
// 连接过滤器
avfilter_link(buffersrc_ctx, 0, buffersink_ctx, 0);
avfilter_graph_config(filter_graph, nullptr);
// 读取视频帧并进行缩放
Mat frame;
AVFrame* scaled_frame = av_frame_alloc();
while (av_read_frame(input_ctx, packet) >= 0)
{
if (packet->stream_index == video_stream_index)
{
avcodec_send_packet(codec_ctx, packet);
while (avcodec_receive_frame(codec_ctx, raw_frame) == 0)
{
av_buffersrc_add_frame_flags(buffersrc_ctx, raw_frame, AV_BUFFERSRC_FLAG_KEEP_REF);
while (av_buffersink_get_frame(buffersink_ctx, scaled_frame) == 0)
{
av_image_fill_arrays(scaled_frame->data, scaled_frame->linesize,
frame.data, AV_PIX_FMT_BGR24, dst_width, dst_height, 1);
frame = Mat(dst_height, dst_width, CV_8UC3, scaled_frame->data[0], scaled_frame->linesize[0]);
imshow("Scaled Video", frame);
waitKey(1);
av_frame_unref(scaled_frame);
}
}
}
av_packet_unref(packet);
}
// 释放资源
avfilter_graph_free(&filter_graph);
avcodec_free_context(&codec_ctx);
av_packet_free(&packet);
av_frame_free(&raw_frame);
av_frame_free(&scaled_frame);
avformat_close_input(&input_ctx);
return 0;
}
```
在这个示例中,我们首先使用ffmpeg打开输入视频文件,并获取视频流的信息和索引。然后创建输入流和输出流的上下文,以及buffersrc和scale_cuda过滤器。最后,我们使用av_read_frame()函数读取视频帧,并使用av_buffersrc_add_frame_flags()函数将原始帧发送到buffersrc过滤器,然后使用av_buffersink_get_frame()函数从buffersink过滤器中获取缩放后的帧。最后,我们将缩放后的帧转换为OpenCV的Mat格式,并使用imshow()函数显示到窗口中。
阅读全文
相关推荐
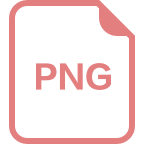
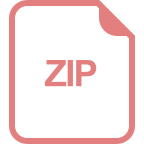
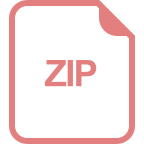
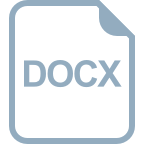
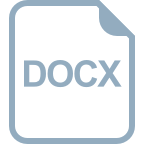