c语言数学函数例子
时间: 2023-08-24 18:23:18 浏览: 68
以下是一些C语言中常见的数学函数的例子:
1. 计算平方根:使用sqrt()函数,例如:
```
#include <math.h>
#include <stdio.h>
int main()
{
double x = 16;
double result = sqrt(x);
printf("The square root of %lf is %lf\n", x, result);
return 0;
}
```
2. 计算绝对值:使用fabs()函数,例如:
```
#include <math.h>
#include <stdio.h>
int main()
{
double x = -3.14;
double result = fabs(x);
printf("The absolute value of %lf is %lf\n", x, result);
return 0;
}
```
3. 计算正弦、余弦和正切值:使用sin()、cos()和tan()函数,例如:
```
#include <math.h>
#include <stdio.h>
int main()
{
double x = 0.5;
double sin_result = sin(x);
double cos_result = cos(x);
double tan_result = tan(x);
printf("sin(%lf) = %lf\n", x, sin_result);
printf("cos(%lf) = %lf\n", x, cos_result);
printf("tan(%lf) = %lf\n", x, tan_result);
return 0;
}
```
4. 计算自然对数和指数函数值:使用log()和exp()函数,例如:
```
#include <math.h>
#include <stdio.h>
int main()
{
double x = 2.718;
double log_result = log(x);
double exp_result = exp(x);
printf("log(%lf) = %lf\n", x, log_result);
printf("exp(%lf) = %lf\n", x, exp_result);
return 0;
}
```
相关推荐
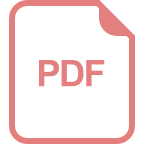
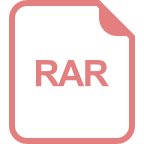
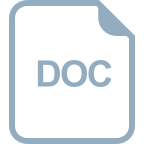














