mybatis如何执行建表命令
时间: 2023-08-14 12:03:31 浏览: 87
在MyBatis中执行建表命令,可以通过两种方式进行操作:使用MyBatis的注解或者使用MyBatis的XML映射文件。
1. 使用注解方式:
- 在实体类上使用`@Table`注解指定表名和字段信息。例如:
```java
@Table(name = "my_table")
public class MyEntity {
@Id
private Long id;
private String name;
// ...
}
```
- 创建一个接口,并使用`@Mapper`注解标记为MyBatis的Mapper接口。在接口中定义建表语句的SQL语句。例如:
```java
@Mapper
public interface MyTableMapper {
@Update("CREATE TABLE my_table (id BIGINT PRIMARY KEY, name VARCHAR(255))")
void createTable();
}
```
- 在Spring Boot应用程序中,注入`MyTableMapper`接口,并在适当的时机调用`createTable()`方法来执行建表语句。例如,在应用程序启动时执行建表语句:
```java
@SpringBootApplication
public class MyApplication implements CommandLineRunner {
private final MyTableMapper myTableMapper;
public MyApplication(MyTableMapper myTableMapper) {
this.myTableMapper = myTableMapper;
}
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
myTableMapper.createTable();
}
}
```
2. 使用XML映射文件方式:
- 创建一个XML映射文件,定义建表语句的SQL语句。例如,在`my_table.xml`中定义建表语句:
```xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.mapper.MyTableMapper">
<update id="createTable">
CREATE TABLE my_table (id BIGINT PRIMARY KEY, name VARCHAR(255))
</update>
</mapper>
```
- 创建一个接口,并使用`@Mapper`注解标记为MyBatis的Mapper接口。在接口中定义一个方法,通过`@Update`注解引用XML映射文件中的建表语句。例如:
```java
@Mapper
public interface MyTableMapper {
@UpdateProvider(type = MyTableSqlProvider.class, method = "createTable")
void createTable();
}
```
- 创建一个SQL提供者类,用于读取XML映射文件中的SQL语句。例如,在`MyTableSqlProvider`中定义一个方法来读取建表语句:
```java
public class MyTableSqlProvider {
public String createTable() {
try {
InputStream inputStream = Resources.getResourceAsStream("my_table.xml");
SqlSessionFactoryBuilder builder = new SqlSessionFactoryBuilder();
SqlSessionFactory factory = builder.build(inputStream);
Configuration configuration = factory.getConfiguration();
MappedStatement statement = configuration.getMappedStatement("com.example.mapper.MyTableMapper.createTable");
BoundSql boundSql = statement.getBoundSql(null);
return boundSql.getSql();
} catch (IOException e) {
throw new RuntimeException("Failed to read SQL from XML file", e);
}
}
}
```
- 在Spring Boot应用程序中,注入`MyTableMapper`接口,并在适当的时机调用`createTable()`方法来执行建表语句。例如,在应用程序启动时执行建表语句:
```java
@SpringBootApplication
public class MyApplication implements CommandLineRunner {
private final MyTableMapper myTableMapper;
public MyApplication(MyTableMapper myTableMapper) {
this.myTableMapper = myTableMapper;
}
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
myTableMapper.createTable();
}
}
```
以上是两种使用MyBatis执行建表命令的常用方式。你可以根据自己的项目需求选择其中一种方式进行操作。
阅读全文
相关推荐
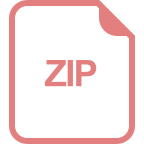

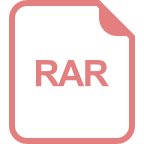
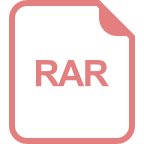
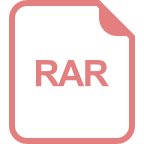
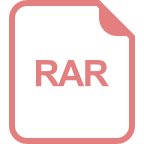
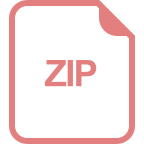
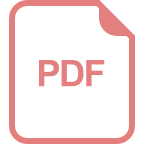
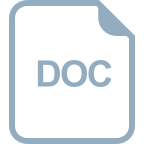
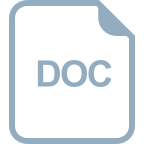
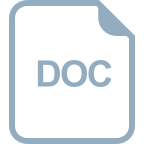
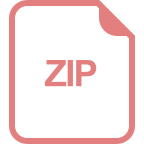
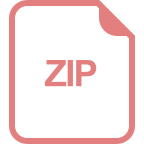
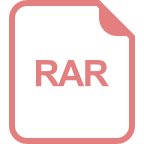
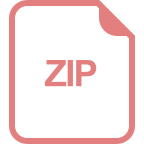