算法在C++程序中被使用时的形式是什么样的
时间: 2024-09-13 13:11:50 浏览: 47
算法是一系列定义明确的操作步骤,用于解决特定的问题或完成特定的任务。在C++程序中,算法通常通过函数(function)或模板函数(template function)的形式被实现和使用。以下是一个简单的例子来说明算法在C++中的使用方式:
```cpp
#include <iostream>
#include <vector>
#include <algorithm> // 包含标准算法头文件
// 自定义算法函数,用于计算向量中的最大值
int findMax(const std::vector<int>& nums) {
if (nums.empty()) {
return -1; // 向量为空时返回-1
}
int maxVal = nums[0];
for (int num : nums) {
if (num > maxVal) {
maxVal = num;
}
}
return maxVal;
}
int main() {
std::vector<int> nums = {1, 3, 5, 7, 9, 2, 4, 6, 8, 0};
int maxVal = findMax(nums);
std::cout << "最大值是: " << maxVal << std::endl;
// 使用标准库算法std::max_element
auto it = std::max_element(nums.begin(), nums.end());
std::cout << "最大值是: " << *it << std::endl;
return 0;
}
```
在上述代码中,`findMax` 函数是一个自定义的算法,它接受一个整型向量,并返回其中的最大值。同时,使用了C++标准库中的 `std::max_element` 算法,该算法直接作用于迭代器,找出给定范围内的最大元素。
在C++中,算法的使用通常遵循这样的模式:
1. 定义算法:可以是独立的函数,也可以是模板函数,以适应不同类型或数据结构的需求。
2. 应用算法:通过调用函数的方式,将数据作为参数传递给算法,执行特定的操作。
3. 利用标准库:C++标准模板库(STL)中提供了丰富的算法,如排序(sort)、搜索(find、search_n、binary_search等)、修改(transform、replace等)、算术(accumulate、inner_product等)等,可以直接使用或进行适当的自定义。
阅读全文
相关推荐
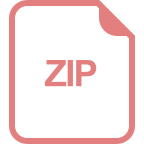
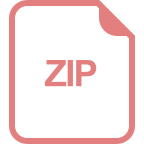
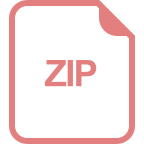
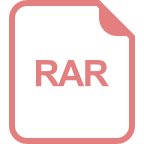
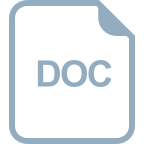
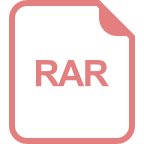
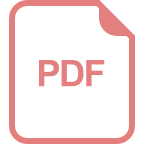
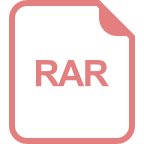
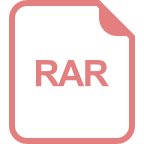
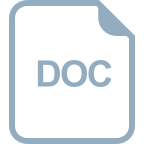
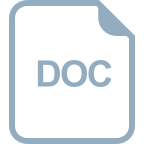
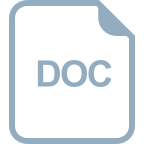
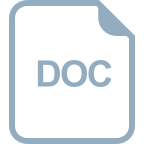
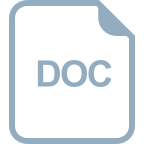
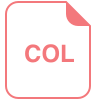
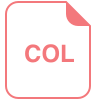
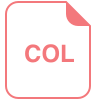
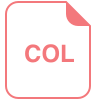