devc++游戏代贪吃蛇
时间: 2023-11-13 20:02:52 浏览: 100
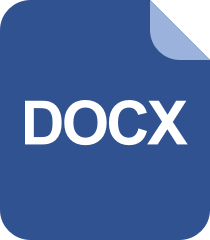
c++代码贪吃蛇游戏直接复制到vc6.0编译器即可.docx
以下是使用DevC++编写的贪吃蛇小游戏的示例代码,其中包括难度选择、计时、加速、暂停等功能:
```c++
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#include <time.h>
#define MAX_X 30
#define MAX_Y 20
#define SNAKE_MAX_LENGTH 100
int map[MAX_X][MAX_Y] = {0}; // 地图
int snake[SNAKE_MAX_LENGTH][2] = {0}; // 蛇
int food[2] = {0}; // 食物
int snake_length = 0; // 蛇的长度
int score = 0; // 得分
int speed = 200; // 速度
int difficulty = 1; // 难度
int is_pause = 0; // 是否暂停
int is_gameover = 0; // 是否游戏结束
// 初始化地图
void init_map() {
int i, j;
for (i = 0; i < MAX_X; i++) {
for (j = 0; j < MAX_Y; j++) {
if (i == 0 || i == MAX_X - 1 || j == 0 || j == MAX_Y - 1) {
map[i][j] = -1; // 边界
} else {
map[i][j] = 0; // 空地
}
}
}
}
// 初始化蛇
void init_snake() {
int i;
snake_length = 3;
for (i = 0; i < snake_length; i++) {
snake[i][0] = MAX_X / 2 + i;
snake[i][1] = MAX_Y / 2;
map[snake[i][0]][snake[i][1]] = i + 1;
}
}
// 初始化食物
void init_food() {
srand((unsigned)time(NULL));
int x, y;
do {
x = rand() % (MAX_X - 2) + 1;
y = rand() % (MAX_Y - 2) + 1;
} while (map[x][y] != 0);
food[0] = x;
food[1] = y;
map[x][y] = -2;
}
// 显示地图
void show_map() {
int i, j;
system("cls");
printf("Score: %d\n", score);
printf("Speed: %d\n", speed);
printf("Difficulty: %d\n", difficulty);
for (i = 0; i < MAX_X; i++) {
for (j = 0; j < MAX_Y; j++) {
switch (map[i][j]) {
case 0:
printf(" ");
break;
case -1:
printf("# ");
break;
case -2:
printf("* ");
break;
default:
printf("o ");
break;
}
}
printf("\n");
}
}
// 移动蛇
void move_snake(int direction) {
int i;
int new_head[2] = {snake[0][0], snake[0][1]};
switch (direction) {
case 1: // 上
new_head[0]--;
break;
case 2: // 下
new_head[0]++;
break;
case 3: // 左
new_head[1]--;
break;
case 4: // 右
new_head[1]++;
break;
}
if (map[new_head[0]][new_head[1]] == -2) { // 吃到食物
snake_length++;
score += 10 * difficulty;
if (speed > 50) {
speed -= 10;
}
init_food();
} else if (map[new_head[0]][new_head[1]] != 0) { // 撞到自己
is_gameover = 1;
return;
}
map[snake[snake_length - 1][0]][snake[snake_length - 1][1]] = 0;
for (i = snake_length - 1; i > 0; i--) {
snake[i][0] = snake[i - 1][0];
snake[i][1] = snake[i - 1][1];
map[snake[i][0]][snake[i][1]] = i + 1;
}
snake[0][0] = new_head[0];
snake[0][1] = new_head[1];
map[new_head[0]][new_head[1]] = 1;
}
// 获取方向
int get_direction() {
int ch = getch();
if (ch == 224) {
ch = getch();
switch (ch) {
case 72: // 上
return 1;
case 80: // 下
return 2;
case 75: // 左
return 3;
case 77: // 右
return 4;
}
} else if (ch == 32) { // 空格键
is_pause = !is_pause;
}
return 0;
}
// 游戏循环
void game_loop() {
int direction = 4;
clock_t last_time = clock();
while (!is_gameover) {
if (!is_pause) {
if (clock() - last_time > speed) {
last_time = clock();
move_snake(direction);
show_map();
}
}
direction = get_direction();
}
printf("Game Over!\n");
}
int main() {
init_map();
init_snake();
init_food();
show_map();
game_loop();
return 0;
}
```
阅读全文
相关推荐
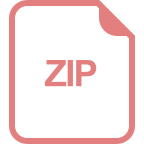
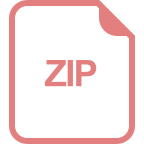
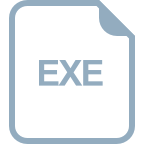
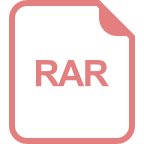
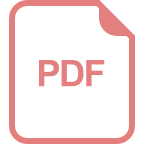
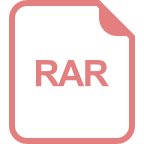
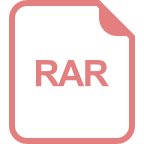
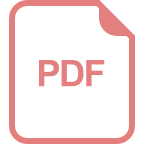
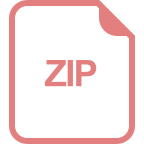
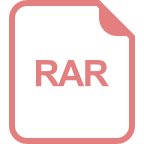