用c语言或c++编写程序,判断一字符串是否是回文。若是回文函数返回值为1;否则返回值为0。
时间: 2024-11-27 15:17:54 浏览: 27
在C语言或C++中,你可以使用双指针法来检查一个字符串是否是回文。这种做法通常涉及两个指针,一个从字符串的开头开始,另一个从字符串的结尾开始,然后逐个比较字符直到它们相遇或者找到不匹配的字符。如果整个过程中所有字符都一一对应相等,则该字符串是回文。
下面是一个简单的C++示例:
```cpp
#include <iostream>
#include <cstring> // for strlen()
bool isPalindrome(const char* str) {
int start = 0;
int end = strlen(str) - 1;
while (start < end) {
if (str[start] != str[end]) {
return false; // 如果找到不相同的字符,立即返回false
}
start++;
end--;
}
return true; // 如果所有字符都匹配,返回true,表示是回文
}
int main() {
std::string testString = "madam";
if (isPalindrome(testString.c_str())) {
std::cout << "The string is a palindrome." << std::endl;
return 1;
} else {
std::cout << "The string is not a palindrome." << std::endl;
return 0;
}
}
```
在这个例子中,`isPalindrome()` 函数接收一个指向字符串的指针,如果字符串是回文则返回1,否则返回0。在`main()` 函数中,我们创建了一个测试字符串并调用了这个函数。
阅读全文
相关推荐
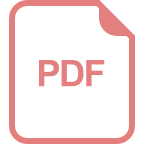
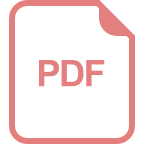
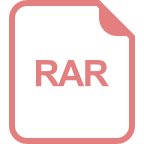

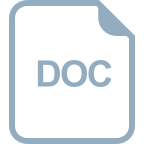
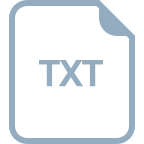
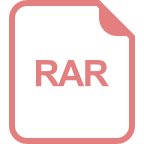
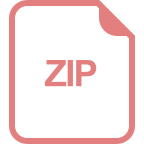
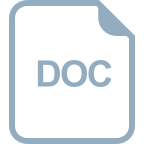
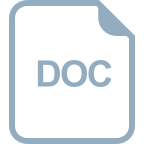
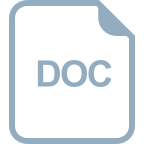


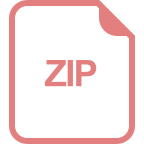
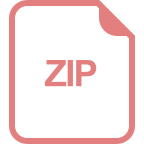
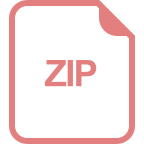
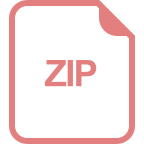
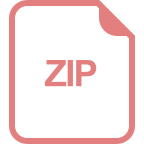