opencv三维重建python
时间: 2023-08-13 18:10:47 浏览: 149
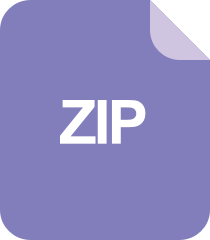
python CT切片图像三维重建(数据和代码).zip
对于使用Python进行OpenCV三维重建,你可以按照以下步骤进行操作:
1. 导入必要的库:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
```
2. 读取图像和标定参数:
```python
img_left = cv2.imread('left_image.jpg')
img_right = cv2.imread('right_image.jpg')
# 左右相机的内参矩阵
K_left = np.array([[fx_left, 0, cx_left],
[0, fy_left, cy_left],
[0, 0, 1]])
K_right = np.array([[fx_right, 0, cx_right],
[0, fy_right, cy_right],
[0, 0, 1]])
# 左右相机的畸变系数
dist_left = np.array([k1_left, k2_left, p1_left, p2_left, k3_left])
dist_right = np.array([k1_right, k2_right, p1_right, p2_right, k3_right])
```
3. 执行立体校正:
```python
# 计算校正映射矩阵
R_left, R_right, P_left, P_right, Q, validPixROI1, validPixROI2 = cv2.stereoRectify(K_left, dist_left,
K_right, dist_right,
(img_width, img_height),
R, T)
# 生成校正映射函数
map_left_x, map_left_y = cv2.initUndistortRectifyMap(K_left, dist_left, R_left,
P_left, (img_width, img_height), cv2.CV_32FC1)
map_right_x, map_right_y = cv2.initUndistortRectifyMap(K_right, dist_right, R_right,
P_right, (img_width, img_height), cv2.CV_32FC1)
# 应用校正映射
img_left_rectified = cv2.remap(img_left, map_left_x, map_left_y, cv2.INTER_LINEAR)
img_right_rectified = cv2.remap(img_right, map_right_x, map_right_y, cv2.INTER_LINEAR)
```
4. 计算视差图:
```python
# 创建SGBM视差算法对象
window_size = 3
min_disp = 0
num_disp = 16 * 5
stereo = cv2.StereoSGBM_create(minDisparity=min_disp,
numDisparities=num_disp,
blockSize=window_size)
# 计算视差图
disp = stereo.compute(img_left_rectified, img_right_rectified).astype(np.float32) / 16.0
```
5. 生成三维点云:
```python
# 根据视差图和校正映射生成点云
points_3d = cv2.reprojectImageTo3D(disp, Q)
# 将点云转换为颜色图像
colors = cv2.cvtColor(img_left_rectified, cv2.COLOR_BGR2RGB)
mask = disp > disp.min()
output_points = points_3d[mask]
output_colors = colors[mask]
# 可选:保存点云数据
output_file = open('point_cloud.ply', 'w')
output_file.write('ply\n')
output_file.write('format ascii 1.0\n')
output_file.write('element vertex %d\n' % output_points.shape[0])
output_file.write('property float x\n')
output_file.write('property float y\n')
output_file.write('property float z\n')
output_file.write('property uchar red\n')
output_file.write('property uchar green\n')
output_file.write('property uchar blue\n')
output_file.write('end_header\n')
for i in range(output_points.shape[0]):
output_file.write('%f %f %f %d %d %d\n' % (
output_points[i, 0], output_points[i, 1], output_points[i, 2],
output_colors[i, 0], output_colors[i, 1], output_colors[i, 2]))
output_file.close()
```
这些步骤可以帮助你使用Python进行OpenCV的三维重建。请确保你已经提供了正确的图像和标定参数,并根据实际情况进行调整。
阅读全文
相关推荐
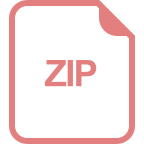
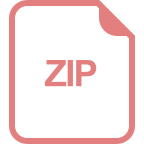















