用C++以班级学期成绩为例,用数组作为线性表存储结构实现 //1.以数组为线性表的存储结构 //a.读成绩表完成构造函数 //b.按某门课程成绩排序 //c.新来的一位同学插入到指定同学的前面或者后面 //d.删除某位同学 //e.修改某位同学的某门课程的成绩
时间: 2024-12-16 22:21:35 浏览: 28
在C++中,我们可以使用数组来实现一个简单的线性表,例如班级学期成绩管理。首先,我们创建一个结构体`Student`表示每位同学的信息,包括姓名和各个科目的成绩。然后,通过数组`students`来存储这个结构体。
```cpp
#include <iostream>
#include <string>
using namespace std;
// 定义学生信息结构
struct Student {
string name;
int scores[5]; // 假设最多5门课程
};
class GradeBook {
private:
int numStudents; // 当前学生数
Student students[];
public:
// 构造函数,读取成绩表并初始化数组
GradeBook(int capacity) {
numStudents = 0;
for (int i = 0; i < capacity && cin >> students[i].name; ++i) {
for (int j = 0; j < 5; ++j) {
cin >> students[i].scores[j];
}
}
}
// 按一门课程成绩排序
void sortByCourse(int courseIndex, bool ascending = true) {
if (courseIndex >= 0 && courseIndex < 5) {
stable_sort(students, students + numStudents, [courseIndex](const Student& a, const Student& b) -> bool {
return ascending ? a.scores[courseIndex] - b.scores[courseIndex] : b.scores[courseIndex] - a.scores[courseIndex];
});
} else {
cout << "Invalid course index.\n";
}
}
// 插入新的同学
void insert(Student newStudent, int position = -1) {
if (position == -1 || position <= numStudents) {
students[numStudents++] = newStudent;
} else {
cout << "Invalid insertion position.\n";
}
}
// 删除一位同学
void remove(int studentIndex) {
if (studentIndex >= 0 && studentIndex < numStudents) {
memmove(&students[studentIndex], &students[studentIndex + 1], sizeof(Student) * (numStudents - 1));
numStudents--;
} else {
cout << "Invalid student index.\n";
}
}
// 修改成绩
void updateScore(int studentIndex, int courseIndex, int newScore) {
if (studentIndex >= 0 && studentIndex < numStudents && courseIndex >= 0 && courseIndex < 5) {
students[studentIndex].scores[courseIndex] = newScore;
} else {
cout << "Invalid operation.\n";
}
}
};
int main() {
GradeBook gradeBook(10); // 创建一个容量为10的学生列表
// 使用gradeBook类的方法操作...
return 0;
}
```
阅读全文
相关推荐





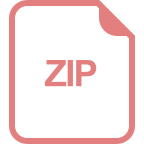










