用C++编写一个顺序表程序
时间: 2024-09-29 12:08:57 浏览: 36
在C++中,我们可以创建一个简单的顺序表(也叫线性表),通常通过数组实现。这里是一个基本的单链表顺序表(Array-Based Linear List)的例子,它包含一个动态大小的数组用于存储元素:
```cpp
#include <iostream>
using namespace std;
// 定义顺序表节点结构体
struct Node {
int data;
Node* next; // 指向下一个节点的指针,对于数组列表为空时设为nullptr
};
// 顺序表类
class SequentialList {
private:
Node* head; // 表头指针,初始指向nullptr
int capacity; // 初始容量
int size; // 当前元素的数量
public:
SequentialList(int initial_capacity = 5) : capacity(initial_capacity), size(0), head(nullptr) {}
// 添加元素到列表尾部
void add(int value) {
if (size == capacity) { // 如果已满,扩大容量
Node* old_head = head;
capacity *= 2; // 新容量为旧容量的两倍
head = new Node[capacity]; // 分配新的内存
for (Node* node = old_head; node != nullptr; node = node->next) {
head[size++] = node->data;
node->next = head + size; // 更新新节点的next指针
}
delete[] old_head; // 释放原内存
} else {
head[size++] = value; // 空闲空间直接添加元素
}
}
// 打印顺序表
void display() const {
Node* current = head;
while (current != nullptr) {
cout << current->data << " ";
current = current->next;
}
cout << endl;
}
};
int main() {
SequentialList list(3);
list.add(1);
list.add(2);
list.add(3);
list.display(); // 输出: 1 2 3
return 0;
}
```
这个例子展示了如何创建一个可以动态扩容的基本顺序表,并实现了添加和打印元素的功能。然而,这只是一个基础版本,实际应用中可能还需要处理更多边界情况和错误检查。
阅读全文
相关推荐
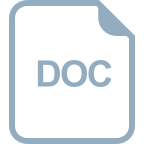
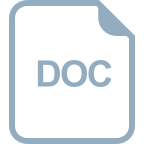
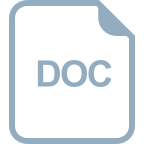
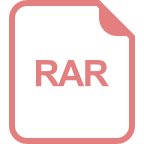

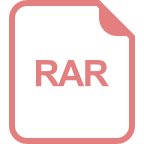
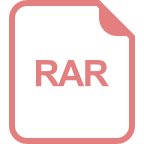
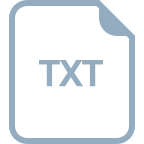








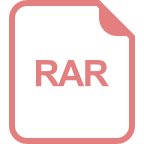
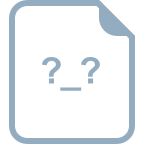