def 2Dto3Dpts(point2D, rVec, tVec, cameraMat, height): """ Function used to convert given 2D points back to real-world 3D points point2D : An array of 2D points rVec : Rotation vector tVec : Translation vector cameraMat: Camera Matrix used in solvePnP height : Height in real-world 3D space Return : output_array: Output array of 3D points """ point3D = [] point2D = (np.array(point2D, dtype='float32')).reshape(-1, 2) numPts = point2D.shape[0] point2D_op = np.hstack((point2D, np.ones((numPts, 1)))) rMat = cv2.Rodrigues(rVec)[0] rMat_inv = np.linalg.inv(rMat) kMat_inv = np.linalg.inv(cameraMat) for point in range(numPts): uvPoint = point2D_op[point, :].reshape(3, 1) tempMat = np.matmul(rMat_inv, kMat_inv) tempMat1 = np.matmul(tempMat, uvPoint) tempMat2 = np.matmul(rMat_inv, tVec) s = (height + tempMat2[2]) / tempMat1[2] p = tempMat1 * s - tempMat2 point3D.append(p) point3D = (np.array(point3D, dtype='float32')).reshape([-1, 1, 3]) return point3D 请说明这份代码中的参数,应该如何获取
时间: 2024-02-15 21:28:20 浏览: 165
这份代码中的参数及其作用如下:
- point2D:一个包含2D点的数组,表示在2D图像上的点坐标。
- rVec:一个旋转向量,表示相机的旋转姿态。
- tVec:一个平移向量,表示相机的平移姿态。
- cameraMat:相机矩阵,包含相机的内参和畸变系数。
- height:一个标量,表示在真实世界中的高度。
这些参数可以通过调用OpenCV的solvePnP函数来获取。solvePnP函数接受3D点和对应的2D点,以及相机矩阵和畸变系数,计算相机的旋转向量和平移向量。在这个函数之后,可以将得到的旋转向量和平移向量作为输入,使用Rodrigues函数将旋转向量转换为旋转矩阵。相机矩阵和畸变系数可以从相机的标定数据中获取。height可以从真实世界中的测量数据中获取。
阅读全文
相关推荐
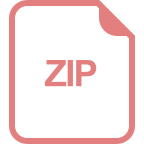
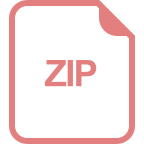
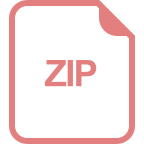
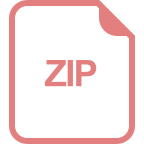
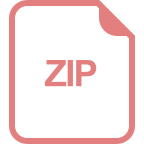
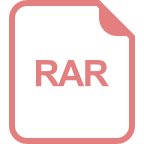
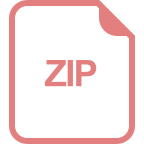
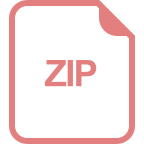
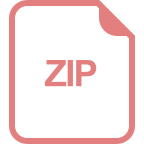
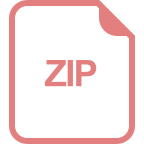
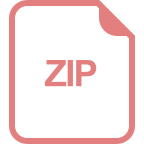
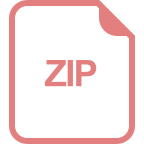
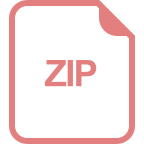
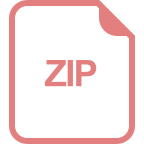
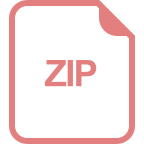
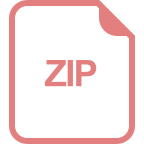
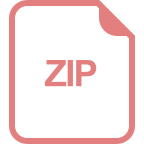