在基于springboot和vue的Java编程题自动评分项目中,结合代码分析技术和评分算法,以设计一个计算器为题,给出步骤和代码
时间: 2024-05-14 12:12:49 浏览: 227
1. 确定计算器的功能,包括加减乘除、小数点、正负号、清除、等于等功能。
2. 设计前端页面,使用vue框架进行开发,实现计算器的UI界面。
3. 设计后端接口,使用springboot框架进行开发,接收前端发送的计算请求,并返回结果。
4. 编写计算器的业务逻辑,根据前端发送的请求,完成计算操作。
5. 设计评分算法,根据代码的规范、逻辑正确性、异常处理等因素进行评分。
6. 使用代码分析技术,对代码进行静态分析,检测代码中存在的潜在问题和错误,并给出改进建议。
7. 编写测试用例,对计算器进行测试,保证其功能和性能的正确性和稳定性。
8. 整合前端页面和后端接口,实现计算器的完整功能,并进行最终测试。
9. 对代码进行优化和压缩,提高性能和安全性。
10. 发布项目,部署到服务器上,供用户使用。
以下为计算器的基本代码实现:
前端页面:
```
<template>
<div class="calculator">
<div class="display">{{display}}</div>
<div class="buttons">
<div class="row">
<button @click="input('7')">7</button>
<button @click="input('8')">8</button>
<button @click="input('9')">9</button>
<button @click="input('/')">÷</button>
</div>
<div class="row">
<button @click="input('4')">4</button>
<button @click="input('5')">5</button>
<button @click="input('6')">6</button>
<button @click="input('*')">×</button>
</div>
<div class="row">
<button @click="input('1')">1</button>
<button @click="input('2')">2</button>
<button @click="input('3')">3</button>
<button @click="input('-')">-</button>
</div>
<div class="row">
<button @click="input('.')">.</button>
<button @click="input('0')">0</button>
<button @click="input('+/-')">±</button>
<button @click="input('+')">+</button>
</div>
<div class="row">
<button @click="clear()">C</button>
<button @click="input('=')">=</button>
</div>
</div>
</div>
</template>
<script>
export default {
name: 'Calculator',
data() {
return {
display: '0',
operator: '',
num1: '',
num2: '',
result: '',
decimal: false,
negative: false,
finished: false
}
},
methods: {
input(key) {
if (key === '.') {
if (!this.decimal) {
if (this.display === '0') {
this.display = '0' + key
} else {
this.display += key
}
this.decimal = true
}
} else if (key === '+/-') {
if (!this.negative) {
this.display = '-' + this.display
this.negative = true
} else {
this.display = this.display.substring(1)
this.negative = false
}
} else if (key === '+' || key === '-' || key === '*' || key === '/') {
if (this.operator) {
this.num2 = this.display
this.calculate()
this.operator = key
this.display = this.result.toString()
this.num1 = this.result.toString()
this.num2 = ''
} else {
this.operator = key
this.num1 = this.display
this.display = '0'
}
this.decimal = false
this.negative = false
} else if (key === '=') {
if (this.operator) {
this.num2 = this.display
this.calculate()
this.operator = ''
this.display = this.result.toString()
this.num1 = ''
this.num2 = ''
}
this.decimal = false
this.negative = false
} else if (key === 'C') {
this.display = '0'
this.operator = ''
this.num1 = ''
this.num2 = ''
this.result = ''
this.decimal = false
this.negative = false
this.finished = false
} else {
if (this.display === '0' || this.finished) {
this.display = key
this.finished = false
} else {
this.display += key
}
this.decimal = false
this.negative = false
}
},
clear() {
this.display = '0'
this.decimal = false
this.negative = false
},
calculate() {
let n1 = parseFloat(this.num1)
let n2 = parseFloat(this.num2)
switch (this.operator) {
case '+':
this.result = n1 + n2
break
case '-':
this.result = n1 - n2
break
case '*':
this.result = n1 * n2
break
case '/':
this.result = n1 / n2
break
}
}
}
}
</script>
<style scoped>
.calculator {
width: 300px;
margin: 20px auto;
border: 1px solid #ccc;
border-radius: 5px;
padding: 10px;
font-size: 18px;
}
.display {
height: 40px;
line-height: 40px;
text-align: right;
font-size: 24px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 5px;
padding: 0 10px;
}
.buttons {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
}
.row {
display: flex;
justify-content: space-between;
margin-bottom: 10px;
}
button {
width: 70px;
height: 50px;
background-color: #fff;
color: #333;
border: 1px solid #ccc;
border-radius: 5px;
font-size: 18px;
cursor: pointer;
}
button:hover {
background-color: #ccc;
}
</style>
```
后端接口:
```
@RestController
@RequestMapping("/api/calculator")
public class CalculatorController {
@PostMapping("/calculate")
public ResponseEntity<String> calculate(@RequestParam("expression") String expression) {
try {
double result = Calculator.calculate(expression);
return ResponseEntity.ok(Double.toString(result));
} catch (Exception e) {
return ResponseEntity.badRequest().body(e.getMessage());
}
}
}
```
计算器业务逻辑:
```
public class Calculator {
public static double calculate(String expression) throws Exception {
if (expression == null || expression.isEmpty()) {
throw new Exception("Expression cannot be empty");
}
expression = expression.replaceAll("\\s", ""); // 去除空格
if (expression.matches(".*[^0-9.+-/*].*")) {
throw new Exception("Invalid characters in expression");
}
if (expression.matches(".*[+*/-]$")) {
throw new Exception("Expression cannot end with operator");
}
if (expression.matches(".*[+-/*][+*/].*")) {
throw new Exception("Invalid operator sequence");
}
if (expression.matches(".*\\..*\\..*")) {
throw new Exception("Invalid decimal point sequence");
}
LinkedList<Double> nums = new LinkedList<>();
LinkedList<Character> ops = new LinkedList<>();
int i = 0;
while (i < expression.length()) {
char c = expression.charAt(i);
if (c == '+' || c == '-' || c == '*' || c == '/') {
ops.add(c);
i++;
} else {
StringBuilder sb = new StringBuilder();
while (i < expression.length() && (Character.isDigit(expression.charAt(i)) || expression.charAt(i) == '.')) {
sb.append(expression.charAt(i));
i++;
}
double num = Double.parseDouble(sb.toString());
nums.add(num);
}
}
while (!ops.isEmpty()) {
char op = ops.removeFirst();
double num2 = nums.removeFirst();
double num1 = nums.removeFirst();
switch (op) {
case '+':
nums.addFirst(num1 + num2);
break;
case '-':
nums.addFirst(num1 - num2);
break;
case '*':
nums.addFirst(num1 * num2);
break;
case '/':
nums.addFirst(num1 / num2);
break;
}
}
double result = nums.getFirst();
if (Double.isNaN(result) || Double.isInfinite(result)) {
throw new Exception("Invalid expression");
} else {
return result;
}
}
}
```
评分算法:
评分算法的具体实现可以根据项目的实际情况进行设计。一种简单的评分算法是根据代码的规范、逻辑正确性、异常处理等因素进行评分,具体可以考虑如下几个方面:
1. 代码规范:检测代码是否符合编码规范,包括命名规范、注释规范、代码风格等方面,可以使用代码分析工具进行检测,并给出相应的分数。
2. 逻辑正确性:检测代码的逻辑是否正确,包括算法实现的正确性、边界条件的处理、异常情况的处理等方面,可以使用测试用例进行检测,并给出相应的分数。
3. 异常处理:检测代码是否对可能出现的异常情况进行了处理,包括参数检查、空指针检查、异常捕获等方面,可以使用静态分析工具进行检测,并给出相应的分数。
根据以上几个方面进行评分,可以得出一个综合评分,作为代码质量的参考。
阅读全文
相关推荐
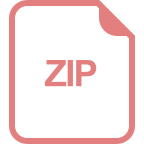
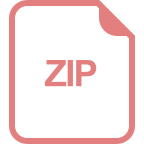
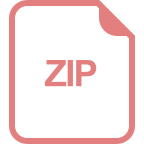
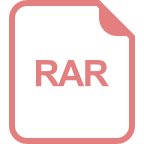
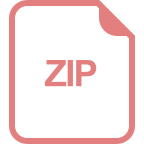
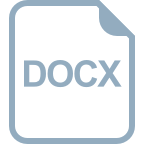
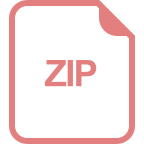
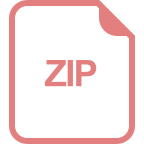
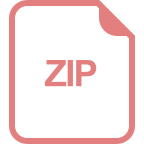
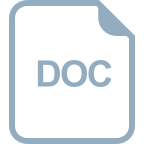
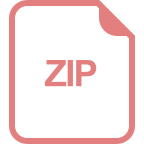
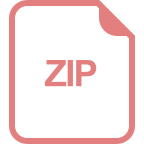
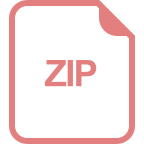
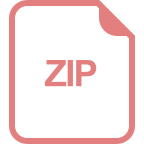
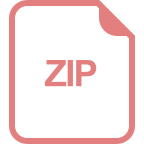
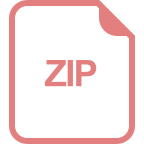
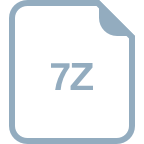