ajax的url不能调用controller层
时间: 2023-09-27 21:12:07 浏览: 50
在MVC架构中,Controller层负责处理请求并返回响应。当使用Ajax时,可以通过Ajax发送请求到Controller中的某个方法,并获取响应。在进行Ajax请求时,需要在url中指定Controller中的方法名,而不是直接调用Controller层。
例如,如果有一个UserController,其中有一个方法getUserById()用于返回用户信息,那么在Ajax请求中应该这样写:
```
$.ajax({
url: "/user/getUserById",
method: "GET",
data: { userId: 123 },
success: function(response) {
// 处理响应
},
error: function(error) {
// 处理错误
}
});
```
这样就可以通过Ajax请求调用UserController中的getUserById()方法,并获取响应了。
相关问题
js如何调用controller中的接口
在前端中,可以使用 Ajax 或者 Fetch API 来调用后端 controller 中的接口。
Ajax 示例代码:
```javascript
$.ajax({
type: 'GET', // 请求方法
url: '/api/user', // 接口地址
dataType: 'json', // 返回数据类型
success: function (data) { // 成功回调函数
console.log(data);
},
error: function (xhr, type) { // 失败回调函数
console.log('请求失败');
}
});
```
Fetch API 示例代码:
```javascript
fetch('/api/user')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
```
以上示例代码中,`/api/user` 是后端控制器中的接口地址,可以根据实际情况进行修改。另外,`$.ajax` 和 `fetch` 方法还有许多可选参数,可以根据实际需求进行设置。
ajax发送请求到controller的代码
以下是一个使用jQuery的示例代码,可以将ajax请求发送到controller:
$.ajax({
url: "/controller/action",
type: "GET",
data: {
param1: "value1",
param2: "value2"
},
success: function(response) {
// 处理成功响应
},
error: function(xhr, status, error) {
// 处理错误响应
}
});
在这个例子中,ajax请求将被发送到名为"action"的controller的URL "/controller"。请求类型为GET,并且包含两个参数"param1"和"param2",它们的值分别为"value1"和"value2"。如果请求成功,将调用成功回调函数,并将响应作为参数传递。如果请求失败,将调用错误回调函数,并将响应状态、错误类型和错误信息作为参数传递。
相关推荐
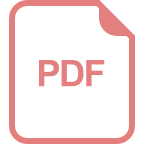
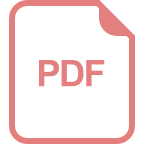
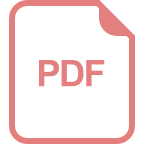












