请使用纯C代码生成CSR文件
时间: 2024-03-21 19:42:53 浏览: 16
生成CSR文件需要使用OpenSSL库提供的函数。以下是使用纯C代码生成CSR文件的示例:
```c
#include <openssl/rsa.h>
#include <openssl/evp.h>
#include <openssl/x509.h>
#include <openssl/pem.h>
int main() {
// 生成RSA密钥对
RSA* rsa = RSA_generate_key(2048, RSA_F4, NULL, NULL);
if (rsa == NULL) {
printf("Failed to generate RSA key pair.\n");
exit(1);
}
// 创建X509证书请求对象
X509_REQ* req = X509_REQ_new();
if (req == NULL) {
printf("Failed to create X509 request object.\n");
exit(1);
}
// 设置X509证书请求的版本号
X509_REQ_set_version(req, 0L);
// 设置X509证书请求的主题名称
X509_NAME* name = X509_NAME_new();
X509_NAME_add_entry_by_txt(name, "CN", MBSTRING_ASC, "example.com", -1, -1, 0);
X509_REQ_set_subject_name(req, name);
// 设置X509证书请求的公钥
EVP_PKEY* pkey = EVP_PKEY_new();
EVP_PKEY_assign_RSA(pkey, rsa);
X509_REQ_set_pubkey(req, pkey);
// 签名X509证书请求
if (!X509_REQ_sign(req, pkey, EVP_sha256())) {
printf("Failed to sign X509 request.\n");
exit(1);
}
// 将X509证书请求保存到文件
FILE* fp = fopen("example.csr", "wb");
if (fp == NULL) {
printf("Failed to create CSR file.\n");
exit(1);
}
PEM_write_X509_REQ(fp, req);
fclose(fp);
// 释放资源
X509_REQ_free(req);
EVP_PKEY_free(pkey);
RSA_free(rsa);
return 0;
}
```
上述代码使用RSA算法生成2048位密钥对,创建X509证书请求并设置主题名称和公钥,最后使用SHA256算法对证书请求进行签名,并将证书请求保存到文件中。注意,这里只是一个简单示例,实际应用中还需要设置更多的证书请求属性。
相关推荐
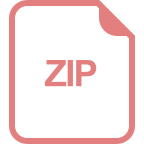














