android实现如何将一个fragment放入一个弹窗的小窗口并放在屏幕中央,这个弹窗实现在preference点击事件里面,弹窗上方增加两个居中Textview,最下方一个确定按钮一个取消按钮;
时间: 2024-03-26 19:41:27 浏览: 15
要实现将一个Fragment放入一个弹窗的小窗口并放在屏幕中央,可以通过使用DialogFragment来实现。具体步骤如下:
1. 创建一个继承自DialogFragment的类,例如MyDialogFragment。
2. 在MyDialogFragment类中,创建一个静态方法newInstance,用于传递参数并创建MyDialogFragment实例。
3. 在MyDialogFragment类中,重写onCreateView方法,将需要放入弹窗的Fragment加载到弹窗中。
4. 在MyDialogFragment类中,重写onActivityCreated方法,设置弹窗的一些属性,例如弹窗的大小、位置等。
5. 在Preference点击事件的回调函数中,创建MyDialogFragment实例,并调用show方法显示弹窗。
6. 在MyDialogFragment中,添加两个TextView和两个Button,并设置它们的属性,例如文字、颜色、大小等。
以下是示例代码:
```java
public class MyDialogFragment extends DialogFragment {
private static final String ARG_PARAM_TITLE = "title";
private static final String ARG_PARAM_MESSAGE = "message";
private static final String ARG_PARAM_CANCELABLE = "cancelable";
private String mTitle;
private String mMessage;
private boolean mCancelable;
public MyDialogFragment() {
// Required empty public constructor
}
public static MyDialogFragment newInstance(String title, String message, boolean cancelable) {
MyDialogFragment fragment = new MyDialogFragment();
Bundle args = new Bundle();
args.putString(ARG_PARAM_TITLE, title);
args.putString(ARG_PARAM_MESSAGE, message);
args.putBoolean(ARG_PARAM_CANCELABLE, cancelable);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
mTitle = getArguments().getString(ARG_PARAM_TITLE);
mMessage = getArguments().getString(ARG_PARAM_MESSAGE);
mCancelable = getArguments().getBoolean(ARG_PARAM_CANCELABLE);
}
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_dialog, container, false);
// 加载需要放入弹窗的Fragment
Fragment fragment = new MyFragment();
getChildFragmentManager().beginTransaction()
.replace(R.id.container, fragment)
.commit();
return view;
}
@Override
public void onActivityCreated(Bundle savedInstanceState) {
super.onActivityCreated(savedInstanceState);
// 设置弹窗的属性
if (getDialog() != null) {
getDialog().getWindow().requestFeature(Window.FEATURE_NO_TITLE);
getDialog().setCancelable(mCancelable);
getDialog().setCanceledOnTouchOutside(mCancelable);
getDialog().getWindow().setLayout(
WindowManager.LayoutParams.MATCH_PARENT,
WindowManager.LayoutParams.WRAP_CONTENT);
getDialog().getWindow().setGravity(Gravity.CENTER);
}
}
}
```
在Preference点击事件的回调函数中,创建MyDialogFragment实例,并调用show方法显示弹窗:
```java
Preference preference = findPreference("key");
preference.setOnPreferenceClickListener(new Preference.OnPreferenceClickListener() {
@Override
public boolean onPreferenceClick(Preference preference) {
MyDialogFragment dialog = MyDialogFragment.newInstance("Title", "Message", true);
dialog.show(getFragmentManager(), "dialog");
return true;
}
});
```
fragment_dialog布局文件中添加两个TextView和两个Button,并设置它们的属性,例如文字、颜色、大小等:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/title_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Title"
android:textColor="@android:color/black"
android:textSize="20sp"
android:layout_gravity="center_horizontal"/>
<TextView
android:id="@+id/message_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Message"
android:textColor="@android:color/black"
android:textSize="16sp"
android:layout_gravity="center_horizontal"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center">
<Button
android:id="@+id/confirm_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Confirm"
android:textSize="16sp"/>
<Button
android:id="@+id/cancel_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Cancel"
android:textSize="16sp"
android:layout_marginLeft="16dp"/>
</LinearLayout>
</LinearLayout>
```
相关推荐
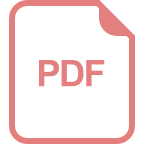
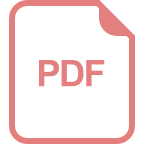
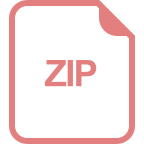














