matlab 生成7位bit哈明码
时间: 2023-11-03 18:15:00 浏览: 92
以下是使用 MATLAB 生成 (7,4) 汉明码的代码示例:
```matlab
% 输入数据位
data = [1 0 1 1];
% 计算校验位
p1 = mod(data(1) + data(2) + data(4), 2);
p2 = mod(data(1) + data(3) + data(4), 2);
p3 = mod(data(2) + data(3) + data(4), 2);
% 构造哈明码
hamming_code = [data p1 p2 data(1) p3 data(2:4)];
% 显示结果
disp("输入数据位: ")
disp(data)
disp("生成的哈明码: ")
disp(hamming_code)
```
在这个示例中,我们首先定义输入的 4 位数据 `data` 为 `[1 0 1 1]`。然后,我们计算出 3 个校验位 `p1`、`p2` 和 `p3`,并将它们插入到哈明码中。最后,我们构造了完整的哈明码,并将其显示出来。
这个示例中的哈明码是一个长度为 7 的二进制码字,其中最后三位是校验位,前四位是数据位。这是一个 (7,4) 汉明码,可以用于检测和纠正单个比特错误。
相关问题
python 实现上位机加密; 1、打开上位机时判断本地是否存在注册文件; 2、若不存在注册文件,则获取C盘序列号和当前日期,组合加密生成明码; 3、将明码解密,将明码中的日期增加30天获得一个停止日期,将停止日期+明码解密出来的C盘序列号+自定义的字符串加密生成密码; 3、上位机输入密码后,根据密码生成一个注册码放在本地生成的一个txt文件; 4、上位机每次打开与注册文件内的注册码进行比较:解密注册文件中的注册码,判断其中的C盘序列号是否与当前的C盘序列号一致,若一致,则判断当前日期是否已超过停止日期,若未超过则可正常打开上位机;
这个问题涉及到了加密解密、文件操作、系统信息获取等多方面的知识。我来简单介绍一下实现的思路:
1. 判断本地是否存在注册文件
可以使用 Python 的 os 模块检查文件是否存在:
```python
import os
if os.path.exists('register.txt'):
# 注册文件存在
else:
# 注册文件不存在,需要生成注册码
```
2. 生成明码
可以使用 Python 内置的 hashlib 模块对 C 盘序列号和当前日期进行哈希加密,生成一个明码:
```python
import hashlib
import datetime
import platform
def get_serial_number():
if platform.system() == 'Windows':
return os.popen('vol C:').read().strip().split()[-1]
elif platform.system() == 'Linux':
return os.popen('blkid -o value -s UUID /dev/sda1').read().strip()
else:
return None
def generate_plain_text():
serial_number = get_serial_number()
date_str = datetime.datetime.now().strftime('%Y-%m-%d')
plain_text = f'{serial_number}-{date_str}'
return plain_text
def generate_hash():
plain_text = generate_plain_text()
hash_obj = hashlib.md5()
hash_obj.update(plain_text.encode())
return hash_obj.hexdigest()
```
3. 生成密码
将生成的明码进行解密,将日期增加 30 天获得一个停止日期,将停止日期、C 盘序列号和自定义字符串组合起来进行加密生成密码:
```python
def generate_password():
hash_str = generate_hash()
stop_date = (datetime.datetime.now() + datetime.timedelta(days=30)).strftime('%Y-%m-%d')
password_plain_text = f'{stop_date}-{get_serial_number()}-my_secret_key'
password_hash_obj = hashlib.md5()
password_hash_obj.update(password_plain_text.encode())
password_hash = password_hash_obj.hexdigest()
return password_hash
```
4. 生成注册文件
将生成的密码写入注册文件:
```python
def generate_register_file():
password_hash = generate_password()
with open('register.txt', 'w') as f:
f.write(password_hash)
```
5. 检查注册文件
每次打开上位机时,先检查注册文件是否存在,如果存在则读取其中的密码进行比较:
```python
def check_register_file():
if not os.path.exists('register.txt'):
return False
with open('register.txt', 'r') as f:
password_hash = f.read().strip()
return password_hash == generate_password()
```
这样就可以实现一个简单的上位机加密功能了。当然,这只是一个简单的示例,实际应用中还需要考虑更多的安全性和可靠性问题。
python实现软件加密 1、首次打开上位机,上位机获取C盘序列号和当前时间组合加密生成明码; 2、客户将明码发回开发者,开发者生成密码;密码可根据发挥的时间设置上位机可用实现;3、生成的密码发给客户 3、客户输入密码后,上位机自动生成一个注册文件;文件包含硬件信息、时限、密码; 4、后需上位机每次打开与注册文件进行校对: 1)硬件信息符合;2)时限不为0;3)密码格式正确;
实现软件加密的一种方法是使用 Python 的加密库,如 PyCrypto。以下是实现步骤:
1. 首次打开上位机,获取 C 盘序列号和当前时间,并将二者组合加密生成明码。
```python
import hashlib
import uuid
import time
# 获取 C 盘序列号
c_drive = uuid.getnode()
# 获取当前时间戳
timestamp = int(time.time())
# 组合成字符串
data = f'{c_drive}{timestamp}'
# 使用 SHA256 加密
hash_object = hashlib.sha256(data.encode())
encrypted_code = hash_object.hexdigest()
```
2. 将明码发回开发者,开发者根据明码生成密码。密码可根据设定的时间范围实现上位机可用的功能。
```python
# 假设开发者设定密码有效期为 30 天
valid_days = 30
# 计算密码失效的时间戳
expiration_timestamp = timestamp + valid_days * 24 * 60 * 60
# 将明码和失效时间戳组合成字符串
data = f'{encrypted_code}{expiration_timestamp}'
# 使用 AES 加密
# 假设开发者使用的是 PyCrypto 库
from Crypto.Cipher import AES
key = b'secretkey123456' # 密钥需要事先协商好
cipher = AES.new(key, AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(data.encode())
password = ciphertext.hex()
```
3. 将生成的密码发给客户。
4. 客户输入密码后,上位机自动生成一个注册文件,包含硬件信息、时限和密码。后续上位机每次打开时,会与注册文件进行校对:
1)硬件信息符合;
2)时限不为0;
3)密码格式正确。
```python
# 假设客户输入的密码为 password_input
# 假设硬件信息和失效时间戳从注册文件中读取
# 将硬件信息和失效时间戳组合成字符串
data = f'{c_drive}{expiration_timestamp}'
# 使用 AES 解密
ciphertext = bytes.fromhex(password_input)
cipher = AES.new(key, AES.MODE_EAX, nonce=nonce)
data_decrypted = cipher.decrypt(ciphertext).decode()
# 检查密码是否正确
if data_decrypted.startswith(encrypted_code):
# 检查时限是否过期
expiration_timestamp_decrypted = int(data_decrypted[len(encrypted_code):])
if expiration_timestamp_decrypted > timestamp:
# 生成注册文件
registration_file = f'{c_drive}_{expiration_timestamp_decrypted}_{password_input}.reg'
else:
print('密码已过期!')
else:
print('密码不正确!')
```
阅读全文
相关推荐
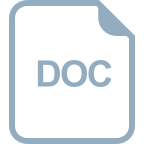
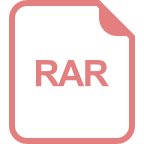
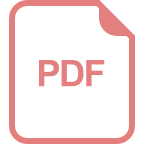
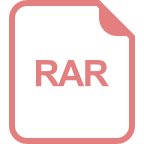
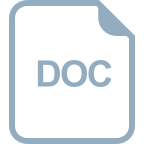



