python和html写代码用来修改mysql表中的内容,并且显示在页面上
时间: 2024-01-24 17:16:54 浏览: 111
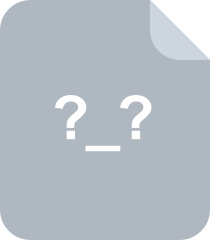
python操作mysql代码
首先,需要安装Python的MySQL驱动程序,例如`mysql-connector-python`。可以使用以下命令安装:
```
pip install mysql-connector-python
```
然后,编写Python代码来连接MySQL数据库,查询和修改表中的内容。例如:
```python
import mysql.connector
# 连接数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
# 查询表中的内容
mycursor = mydb.cursor()
mycursor.execute("SELECT * FROM yourtable")
myresult = mycursor.fetchall()
# 修改表中的内容
sql = "UPDATE yourtable SET yourcolumn = %s WHERE id = %s"
val = ("new value", 1)
mycursor.execute(sql, val)
mydb.commit()
# 关闭数据库连接
mydb.close()
```
接下来,需要使用HTML和Python的web框架(如Flask或Django)来创建一个web应用程序,将查询和修改表中的内容显示在页面上。例如,在Flask中,可以使用以下代码:
```python
from flask import Flask, render_template
import mysql.connector
app = Flask(__name__)
@app.route('/')
def index():
# 连接数据库并查询表中的内容
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
mycursor = mydb.cursor()
mycursor.execute("SELECT * FROM yourtable")
myresult = mycursor.fetchall()
mydb.close()
# 将查询结果传递给HTML模板
return render_template('index.html', result=myresult)
if __name__ == '__main__':
app.run()
```
在HTML模板中,可以使用模板语言(如Jinja2)将查询结果渲染到页面上。例如:
```html
<!DOCTYPE html>
<html>
<head>
<title>MySQL Table</title>
</head>
<body>
<table>
<thead>
<tr>
<th>ID</th>
<th>Column 1</th>
<th>Column 2</th>
</tr>
</thead>
<tbody>
{% for row in result %}
<tr>
<td>{{ row[0] }}</td>
<td>{{ row[1] }}</td>
<td>{{ row[2] }}</td>
</tr>
{% endfor %}
</tbody>
</table>
</body>
</html>
```
这将创建一个包含表中内容的HTML表格。可以在模板中添加表单和Python代码来实现修改表中内容的功能。
阅读全文
相关推荐
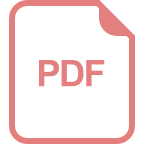

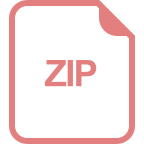
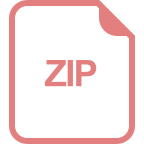
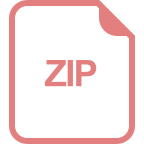
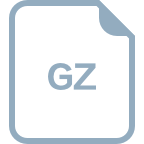
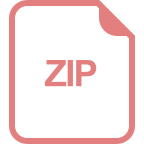
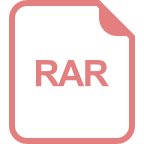
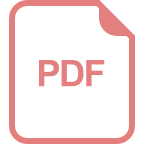
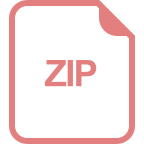
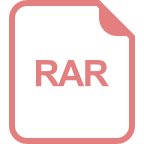
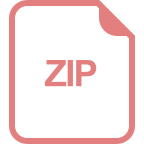
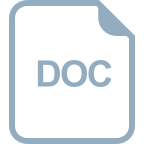
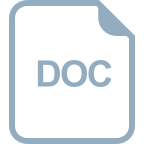
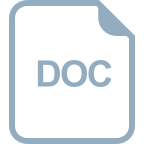
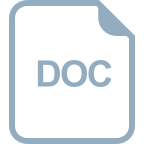
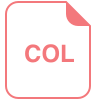