python combobox获取值判断是否为空
时间: 2023-06-14 08:04:07 浏览: 55
在 Python 中使用 tkinter 库可以创建图形界面 (GUI)。其中,Combobox 控件可以在下拉菜单中选择一个值。
要获取 Combobox 控件的当前值,可以使用 `get()` 方法:
```python
selected_value = combobox.get()
```
然后,可以使用 `if` 语句来判断该值是否为空:
```python
if selected_value:
# selected_value 不为空
else:
# selected_value 为空
```
注意,在 Python 中,空值 (empty value) 包括空字符串、None、[]、() 和 {}。所以,如果 Combobox 控件中没有选择任何值,那么 `selected_value` 的值就是一个空字符串。
相关问题
python combobox 每选择一项,则向lineEdit添加被选择combobox项的文本,每次用'、'隔开
可以使用以下代码实现:
```python
from PyQt5.QtWidgets import QApplication, QComboBox, QLineEdit, QVBoxLayout, QWidget
class MyWidget(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.combobox = QComboBox()
self.combobox.addItems(['item1', 'item2', 'item3'])
self.combobox.currentIndexChanged.connect(self.add_text)
self.lineedit = QLineEdit()
layout = QVBoxLayout()
layout.addWidget(self.combobox)
layout.addWidget(self.lineedit)
self.setLayout(layout)
self.show()
def add_text(self, index):
current_text = self.combobox.currentText()
if index != -1 and current_text not in self.lineedit.text():
self.lineedit.setText(self.lineedit.text() + current_text + '、')
if __name__ == '__main__':
app = QApplication([])
widget = MyWidget()
app.exec_()
```
在这个例子中,我们使用了一个 QComboBox 和一个 QLineEdit。每次选择 QComboBox 中的一项,都会触发 currentIndexChanged 信号,然后调用 add_text 函数。在 add_text 函数中,我们获取当前选择的文本,判断这个文本是否已经在 QLineEdit 中出现,如果没有出现,则将这个文本添加到 QLineEdit 中,并在文本末尾加上 '、'。
我在使用pyqt中的tablewidget时,有一个单元的内容是一个combobox控件,当我遍历整个table的内容时,我怎么获取到combox对应的值,给我一个相关实例
当TableWidget中有一个单元的内容是ComboBox控件时,可以通过以下方式获取ComboBox的值:
```python
from PyQt5.QtWidgets import QComboBox, QTableWidgetItem, QTableWidget
tableWidget = QTableWidget()
rowCount = tableWidget.rowCount()
columnCount = tableWidget.columnCount()
for row in range(rowCount):
for column in range(columnCount):
item = tableWidget.item(row, column)
if isinstance(item, QTableWidgetItem):
# 如果单元格中的内容是QTableWidgetItem,则获取其文本信息
value = item.text()
elif isinstance(item, QComboBox):
# 如果单元格中的内容是QComboBox,则获取当前选中的文本信息
value = item.currentText()
else:
value = None
print(value)
```
上述代码中,我们通过遍历TableWidget的每个单元格,判断单元格中的内容是QTableWidgetItem还是QComboBox,然后分别获取其文本信息或当前选中的文本信息。
注意:如果TableWidget中存在多个ComboBox控件,需要根据实际情况进行判断,例如可以通过设置单元格的特定属性来标识哪些单元格是ComboBox控件。
相关推荐
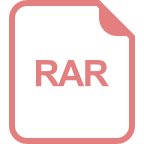
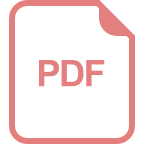
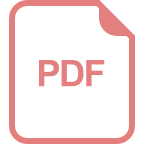












