iOS网络音频url存储到本地播放
时间: 2023-10-03 20:03:17 浏览: 60
要实现将网络音频URL存储到本地并播放,可以按照以下步骤进行操作:
1. 首先,使用AVPlayer或AVPlayerViewController来播放网络音频。例如:
```swift
let url = URL(string: "http://example.com/audio.mp3")
let player = AVPlayer(url: url!)
let playerViewController = AVPlayerViewController()
playerViewController.player = player
present(playerViewController, animated: true) {
player.play()
}
```
2. 接下来,使用URLSession来下载音频文件并将其保存到本地。例如:
```swift
let url = URL(string: "http://example.com/audio.mp3")
let task = URLSession.shared.downloadTask(with: url!) { (location, response, error) in
guard let location = location else { return }
let documentsUrl = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first!
let destinationUrl = documentsUrl.appendingPathComponent("audio.mp3")
do {
try FileManager.default.moveItem(at: location, to: destinationUrl)
// 播放本地音频
let player = AVPlayer(url: destinationUrl)
let playerViewController = AVPlayerViewController()
playerViewController.player = player
present(playerViewController, animated: true) {
player.play()
}
} catch {
print(error)
}
}
task.resume()
```
3. 最后,为了节省空间,可以在不需要时删除本地音频文件。例如:
```swift
let fileManager = FileManager.default
let documentsUrl = fileManager.urls(for: .documentDirectory, in: .userDomainMask).first!
let destinationUrl = documentsUrl.appendingPathComponent("audio.mp3")
do {
try fileManager.removeItem(at: destinationUrl)
} catch {
print(error)
}
```
以上就是实现将网络音频URL存储到本地并播放的步骤。
相关推荐
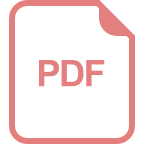
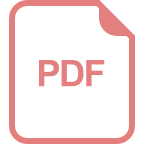
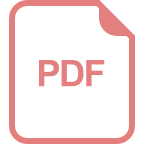














