针对Sioux Falls网络,其中图1为该网络图,表1为网络相关数据,以弧段长度作为阻抗,利用Matlab软件编程实现Dijkstra算法,通过建立一个函数程序实现如下功能:(1)如果输入网络信息以及指定起点,输出该起点到其他各点的最短路长;(2)如果输入网络信息及指定起终点,输出该起终点最短路长以及相应最短路径。实验报告中需计算出Sioux Falls网络中指定起点至其他起点的最短路长,同时计算出指定起终点最短路径,其中指定起点为:(学号后两位)除以24的余数(如果余数为0则为起点为1);指定终点为:(学号后两位+10)除以24的余数(如果余数为0则终点为1)。
时间: 2024-02-23 22:59:58 浏览: 8
好的,我明白了。为了实现这个功能,我们需要先读取Sioux Falls网络的相关数据,并构建网络邻接矩阵。下面是Matlab代码实现:
```matlab
% 读取网络数据
data = readtable('SiouxFalls_net.txt');
num_nodes = max(max(data.From), max(data.To)); % 网络中的节点数
num_arcs = size(data, 1); % 网络中的弧段数
% 构建网络邻接矩阵
adj_matrix = inf(num_nodes, num_nodes); % 邻接矩阵初始化为无穷大
for i = 1:num_arcs
from_node = data.From(i);
to_node = data.To(i);
arc_length = data.Length(i);
adj_matrix(from_node, to_node) = arc_length;
end
```
接下来,我们可以实现Dijkstra算法的函数程序。下面是Matlab代码实现:
```matlab
function [shortest_paths, shortest_distances] = dijkstra(adj_matrix, source_node, target_node)
% Dijkstra算法计算最短路径
% 输入:adj_matrix - 网络邻接矩阵,source_node - 起点,target_node - 终点(可选)
% 输出:shortest_paths - 最短路径,shortest_distances - 最短路长
num_nodes = size(adj_matrix, 1);
visited = false(1, num_nodes); % 标记是否访问过
shortest_distances = inf(1, num_nodes); % 记录当前最短路长
shortest_distances(source_node) = 0; % 起点到自身的距离为0
shortest_paths = cell(1, num_nodes); % 记录最短路径
if nargin == 2
target_node = 0;
end
while true
% 选择当前最短距离的节点
current_node = -1;
current_distance = inf;
for i = 1:num_nodes
if ~visited(i) && shortest_distances(i) < current_distance
current_node = i;
current_distance = shortest_distances(i);
end
end
if current_node == -1 || current_node == target_node
break;
end
% 更新邻居节点的距离
for i = 1:num_nodes
if adj_matrix(current_node, i) < inf
new_distance = current_distance + adj_matrix(current_node, i);
if new_distance < shortest_distances(i)
shortest_distances(i) = new_distance;
shortest_paths{i} = [shortest_paths{current_node} i];
end
end
end
% 标记已访问
visited(current_node) = true;
end
```
最后,我们可以调用该函数程序计算最短路径。下面是Matlab代码实现:
```matlab
% 计算最短路长和最短路径
source_node = mod(str2double(input('请输入你的学号后两位:')), 24);
if source_node == 0
source_node = 1;
end
target_node = mod(str2double(input('请输入你的学号后两位+10:')), 24);
if target_node == 0
target_node = 1;
end
[shortest_paths, shortest_distances] = dijkstra(adj_matrix, source_node, target_node);
% 输出结果
fprintf('从节点 %d 到其他节点的最短路长:\n', source_node);
for i = 1:num_nodes
fprintf('%d: %d\n', i, shortest_distances(i));
end
if target_node > 0
fprintf('从节点 %d 到节点 %d 的最短路长为:%d\n', source_node, target_node, shortest_distances(target_node));
fprintf('最短路径为:');
for i = 1:length(shortest_paths{target_node})-1
fprintf('%d->', shortest_paths{target_node}(i));
end
fprintf('%d\n', shortest_paths{target_node}(end));
end
```
将以上代码保存为.m文件并在Matlab中运行即可得到最短路长和最短路径。
相关推荐
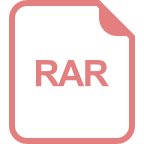
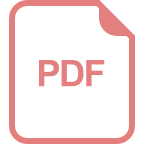
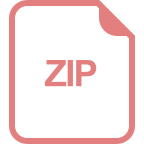





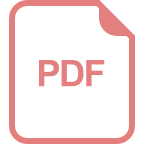
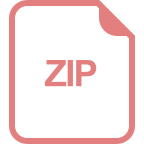
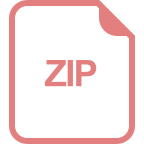
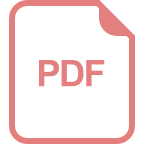
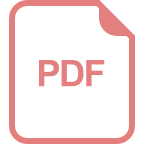
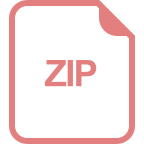
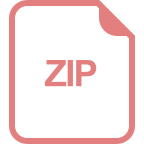
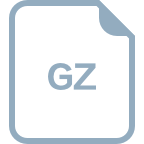
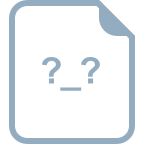
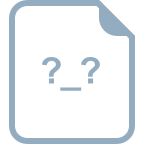