springboot集成redission
时间: 2023-04-11 11:04:57 浏览: 82
Spring Boot集成Redisson可以通过以下步骤实现:
1. 添加Redisson依赖
在pom.xml文件中添加Redisson依赖:
```
<dependency>
<groupId>org.redisson</groupId>
<artifactId>redisson-spring-boot-starter</artifactId>
<version>3.15.5</version>
</dependency>
```
2. 配置Redisson
在application.properties文件中添加Redisson配置:
```
spring.redis.host=127.0.0.1
spring.redis.port=6379
spring.redis.password=
spring.redis.database=0
```
3. 使用Redisson
在需要使用Redisson的地方注入RedissonClient:
```
@Autowired
private RedissonClient redissonClient;
```
然后就可以使用Redisson提供的API进行操作了,例如:
```
RMap<String, String> map = redissonClient.getMap("myMap");
map.put("key", "value");
```
以上就是Spring Boot集成Redisson的简单步骤。
相关问题
springboot 实现redission
Spring Boot可以通过引入Redisson库来实现对Redisson的使用。在Spring Boot中,首先需要配置Redisson的启动类,并指定Redis的连接信息,如主机、端口、密码等。然后,可以通过RedissonClient对象来获取Redisson的实例,从而进行相应的操作。
首先,在Spring Boot的启动类中,需要添加`@SpringBootApplication`注解,并在`main`方法中调用`SpringApplication.run`方法来启动应用。
其次,在配置文件中,需要设置Redis的连接信息,例如主机名、端口号、密码等。这些配置项可以通过`application.properties`或`application.yml`文件进行配置。
然后,在具体的代码中,可以使用`@Resource`注解注入`RedissonClient`对象,并通过该对象获取对应的锁实例。例如,可以使用`getLock`方法来获取一个锁,并使用`tryLock`方法来尝试获取该锁。如果成功获取到锁,则可以进行相应的操作。
需要注意的是,在使用完锁之后,需要调用`unlock`方法来释放锁资源,以避免资源泄漏或死锁等问题。
以上是使用Spring Boot实现Redisson的简单示例,具体的使用方式可以根据实际需求来进行调整和扩展。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [springboot集成redission(代码完整版)](https://blog.csdn.net/m0_37825155/article/details/120696303)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *3* [springboot+redission再相识](https://blog.csdn.net/leijie0322/article/details/126777979)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
springboot中redission依赖
在springboot中使用redission,需要在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.redisson</groupId>
<artifactId>redisson-spring-boot-starter</artifactId>
<version>3.16.0</version>
</dependency>
```
其中,版本号可以根据实际情况进行调整。
相关推荐
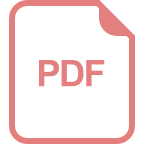
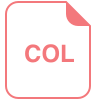
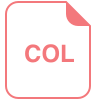
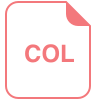
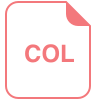
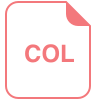







