帮我使用GO的泛型定义一个动画类的代码
时间: 2023-03-29 12:01:34 浏览: 111
好的,以下是使用GO的泛型定义一个动画类的代码:
```
package main
import (
"fmt"
)
type Animation[T any] struct {
frames []T
}
func (a *Animation[T]) AddFrame(frame T) {
a.frames = append(a.frames, frame)
}
func (a *Animation[T]) Play() {
for _, frame := range a.frames {
fmt.Println(frame)
}
}
func main() {
a := Animation[string]{}
a.AddFrame("Frame 1")
a.AddFrame("Frame 2")
a.AddFrame("Frame 3")
a.Play()
}
```
这个动画类使用了GO的泛型,可以接受任何类型的帧。在这个例子中,我们使用了字符串类型作为帧。
相关问题
帮我使用java的泛型定义一个动画类的代码
好的,以下是使用 Java 泛型定义动画类的代码:
```
public class Animation<T> {
private T frames;
public Animation(T frames) {
this.frames = frames;
}
public T getFrames() {
return frames;
}
public void setFrames(T frames) {
this.frames = frames;
}
}
```
其中,泛型类型参数 `T` 表示动画帧的类型,可以是任何类型。在构造函数中,我们将动画帧作为参数传入,并将其保存在私有成员变量 `frames` 中。我们还提供了 `getFrames()` 和 `setFrames()` 方法,用于获取和设置动画帧。
帮我使用ts的泛型定义一个动画类的代码
好的,以下是使用 TypeScript 泛型定义的动画类代码:
```
interface AnimationOptions {
duration: number;
easing: string;
}
class Animation<T> {
private startValue: T;
private endValue: T;
private duration: number;
private easing: string;
constructor(startValue: T, endValue: T, options: AnimationOptions) {
this.startValue = startValue;
this.endValue = endValue;
this.duration = options.duration;
this.easing = options.easing;
}
animate(onUpdate: (value: T) => void, onComplete?: () => void) {
const startTime = Date.now();
const endTime = startTime + this.duration;
const animateFrame = () => {
const now = Date.now();
const progress = Math.min(1, (now - startTime) / this.duration);
const easedProgress = this.easingFunction(progress);
const currentValue = this.interpolate(this.startValue, this.endValue, easedProgress);
onUpdate(currentValue);
if (now < endTime) {
requestAnimationFrame(animateFrame);
} else if (onComplete) {
onComplete();
}
};
requestAnimationFrame(animateFrame);
}
private easingFunction(progress: number) {
// TODO: Implement easing function
return progress;
}
private interpolate(startValue: T, endValue: T, progress: number) {
// TODO: Implement interpolation function
return startValue;
}
}
```
希望这个代码对你有帮助!
相关推荐
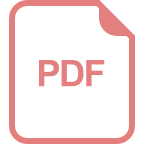
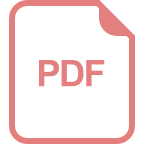
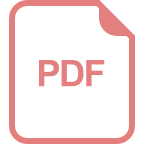












