java 连接 mysql
时间: 2023-07-11 11:38:55 浏览: 93
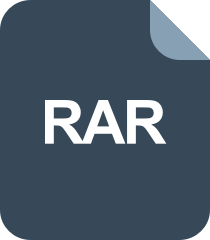
java连接mysql数据库
可以使用 JDBC(Java Database Connectivity)API 来连接 MySQL 数据库。
以下是连接 MySQL 数据库的步骤:
1. 导入 JDBC 驱动程序
在项目中导入 MySQL Connector/J 驱动程序,可以从 MySQL 官方网站下载。
2. 注册 JDBC 驱动程序
使用 Class.forName() 方法来注册驱动程序。例如:
```
Class.forName("com.mysql.jdbc.Driver");
```
3. 建立连接
使用 DriverManager.getConnection() 方法来建立与 MySQL 数据库的连接。例如:
```
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "root";
String password = "password";
Connection conn = DriverManager.getConnection(url, user, password);
```
其中,url 是连接字符串,mydatabase 是要连接的数据库名称,root 是 MySQL 用户名,password 是用户密码。
4. 执行 SQL 查询和更新
使用 Connection 对象的 createStatement() 方法来创建 Statement 对象,然后使用 Statement 对象来执行 SQL 查询和更新。例如:
```
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM mytable");
int rows = stmt.executeUpdate("INSERT INTO mytable (name, age) VALUES ('John', 30)");
```
其中,executeQuery() 方法用于执行 SELECT 查询语句,返回 ResultSet 对象;executeUpdate() 方法用于执行 INSERT、UPDATE 或 DELETE 操作,返回受影响的行数。
5. 关闭连接
使用 Connection 对象的 close() 方法来关闭与 MySQL 数据库的连接。例如:
```
conn.close();
```
完整的 Java 代码示例:
```
import java.sql.*;
public class MySQLTest {
public static void main(String[] args) {
try {
// 导入 JDBC 驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 建立连接
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "root";
String password = "password";
Connection conn = DriverManager.getConnection(url, user, password);
// 执行 SQL 查询
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM mytable");
while (rs.next()) {
System.out.println(rs.getString("name") + ", " + rs.getInt("age"));
}
// 执行 SQL 更新
int rows = stmt.executeUpdate("INSERT INTO mytable (name, age) VALUES ('John', 30)");
System.out.println(rows + " rows affected.");
// 关闭连接
conn.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
阅读全文
相关推荐
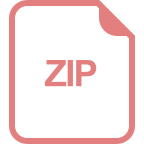
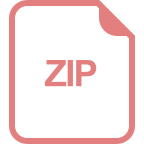



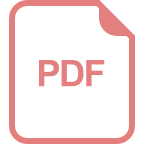
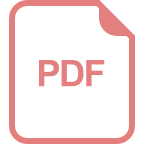
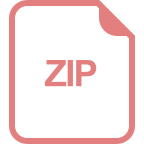
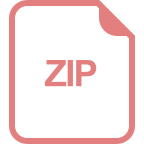
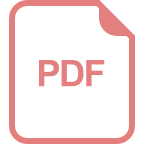
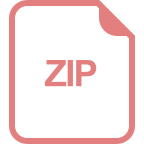